在 Spring Boot 中,Bean 是应用程序的核心组件,它们代表了应用程序中的各种对象,如服务、数据源、配置等。Bean 的加载过程是 Spring Boot 启动时的关键步骤之一,它负责实例化、配置和管理这些 Bean,使它们可以在整个应用程序中被访问和使用。
Bean 加载的主要目的是提供依赖注入和 IoC(控制反转)容器,以便更轻松地管理组件之间的关系,从而使应用程序更加松散耦合和易于维护。
Spring Boot Bean 加载过程
Spring Boot 的 bean 加载过程是 Spring 框架的一部分,它建立在 Spring Framework 之上,并提供了简化的配置和开发方式。Spring Boot 的 bean 加载过程与 Spring 框架的 bean 加载过程类似,但有一些特定的步骤和自动化配置。
以下是 Spring Boot 中 bean 加载的主要步骤:
1、启动应用程序:Spring Boot 应用程序通常通过main
方法启动,其中包含一个包含@SpringBootApplication
注解的类。这个注解标志着这是一个 Spring Boot 应用程序,它会触发自动配置和 bean 加载过程。
2、自动配置:Spring Boot 自动配置机制会根据类路径上的依赖和应用程序的配置文件来自动配置应用程序。它会创建一些常见的 bean,例如DataSource
、EntityManagerFactory
、TransactionManager
等,以及一些通用的配置。
3、扫描组件:Spring Boot 会自动扫描应用程序包及其子包,查找带有@Component
、@Service
、@Repository
等注解的类。这些注解表明类是 Spring 的组件,并且应该被 Spring 容器管理。
4、创建 bean 定义:当 Spring Boot 发现一个带有上述注解的类时,它会创建一个对应的 bean 定义。这个 bean 定义包含有关如何创建和配置 bean 的信息。
5、初始化 bean:Spring Boot 会实例化并初始化 bean。这包括调用构造函数(如果有的话)、设置属性、调用初始化方法(如果有的话)等操作。
6、依赖注入:如果 bean 依赖于其他 bean,Spring Boot 会使用依赖注入机制将这些依赖注入到 bean 中。这是通过在构造函数、setter 方法或字段上使用@Autowired
注解来实现的。
7、Post-Processing(后处理):Spring Boot 还支持 Bean 后处理器。这些后处理器允许你在 bean 初始化之前或之后执行自定义逻辑。你可以使用@PostConstruct
和@PreDestroy
注解定义初始化和销毁回调方法。
8、Bean 就绪:一旦所有 bean 都被初始化和配置,应用程序就准备好运行了。
总的来说,Spring Boot 的 bean 加载过程是自动的、基于注解的,它大大简化了传统的 Spring 应用程序的配置工作。Spring Boot 会根据应用程序的依赖和配置文件自动进行大部分工作,从而使开发者能够更专注于业务逻辑的实现。同时,Spring Boot 也提供了一些可扩展性选项,以便在需要时进行自定义配置和操作。
Spring Boot Bean 配置
要在 Spring Boot 项目中使用 Bean 加载功能,你可以遵循以下步骤:
1、添加依赖:确保你的项目中已添加 Spring Boot 相关的依赖项,包括 Spring Boot Starter。
2、创建 Bean:编写 Java 类,并使用@Component
或其他相关的注解将其标记为 Bean。
3、配置文件:根据需要,在application.properties
或application.yml
中配置 Bean 的属性和值。
4、注入 Bean:在其他组件中使用@Autowired
等注解来注入你创建的 Bean。
实践案例
以下是一个简单的 Spring Boot bean 加载过程的示例,包括如何创建 bean 以及它们如何在应用程序中加载和使用。
假设我们正在构建一个简单的学生管理系统,其中有两个主要的 bean:Student
和StudentService
。
1、创建Student
类:
public class Student {
private Long id;
private String name;
private int age;
// 构造函数、getter和setter方法
}
2、创建StudentService
类:
@Service
public class StudentService {
private List<Student> students = new ArrayList<>();
// 添加学生
public void addStudent(Student student) {
students.add(student);
}
// 获取所有学生
public List<Student> getAllStudents() {
return students;
}
}
在这里,我们使用了@Service
注解来标记StudentService
,这告诉 Spring Boot 它是一个 bean 并应该由 Spring 容器管理。
3、创建 Spring Boot 应用程序主类:
@SpringBootApplication
public class StudentManagementApplication {
public static void main(String[] args) {
SpringApplication.run(StudentManagementApplication.class, args);
}
}
这个主类使用了@SpringBootApplication
注解,它会触发 Spring Boot 的自动配置和 bean 加载过程。
4、使用 StudentService:现在,我们可以在应用程序中使用StudentService
来添加和获取学生。例如,我们可以在控制器中使用它:
@RestController
@RequestMapping("/students")
public class StudentController {
@Autowired
private StudentService studentService;
@PostMapping
public void addStudent(@RequestBody Student student) {
studentService.addStudent(student);
}
@GetMapping
public List<Student> getAllStudents() {
return studentService.getAllStudents();
}
}
这个控制器使用@Autowired
注解来注入StudentService
bean,并使用它来添加和获取学生。
现在,当你启动应用程序时,Spring Boot 会执行以下操作:
- 扫描类路径,发现
StudentService
被@Service
注解标记。 - 创建
StudentService
的 bean 定义。 - 实例化
StudentService
bean。 - 注入
StudentService
到StudentController
。 - 当访问
/students
端点时,StudentController
会使用StudentService
来添加和获取学生。
这是一个简单的示例,演示了 Spring Boot bean 加载过程。在实际应用程序中,你可以有更多的 bean,它们之间可能存在复杂的依赖关系,但 Spring Boot 会负责管理它们,以便你可以专注于应用程序的开发。
使用 Apifox 测试和管理接口
如果你是 JAVA 开发者,你经常需要与 API 打交道,确保你的应用程序能够正常工作。这时,一个强大的接口测试工具就会派上用场。
Apifox 是一个比 Postman 更强大的接口测试工具,Apifox = Postman + Swagger + Mock + JMeter,Apifox 支持调试 http(s)、WebSocket、Socket、gRPC、Dubbo 等协议的接口,并且集成了 IDEA 插件。在开发完接口后,可以通过 Apifox 的 IDEA 插件一键自动生成接口文档,多端同步,非常方便测试和维护。
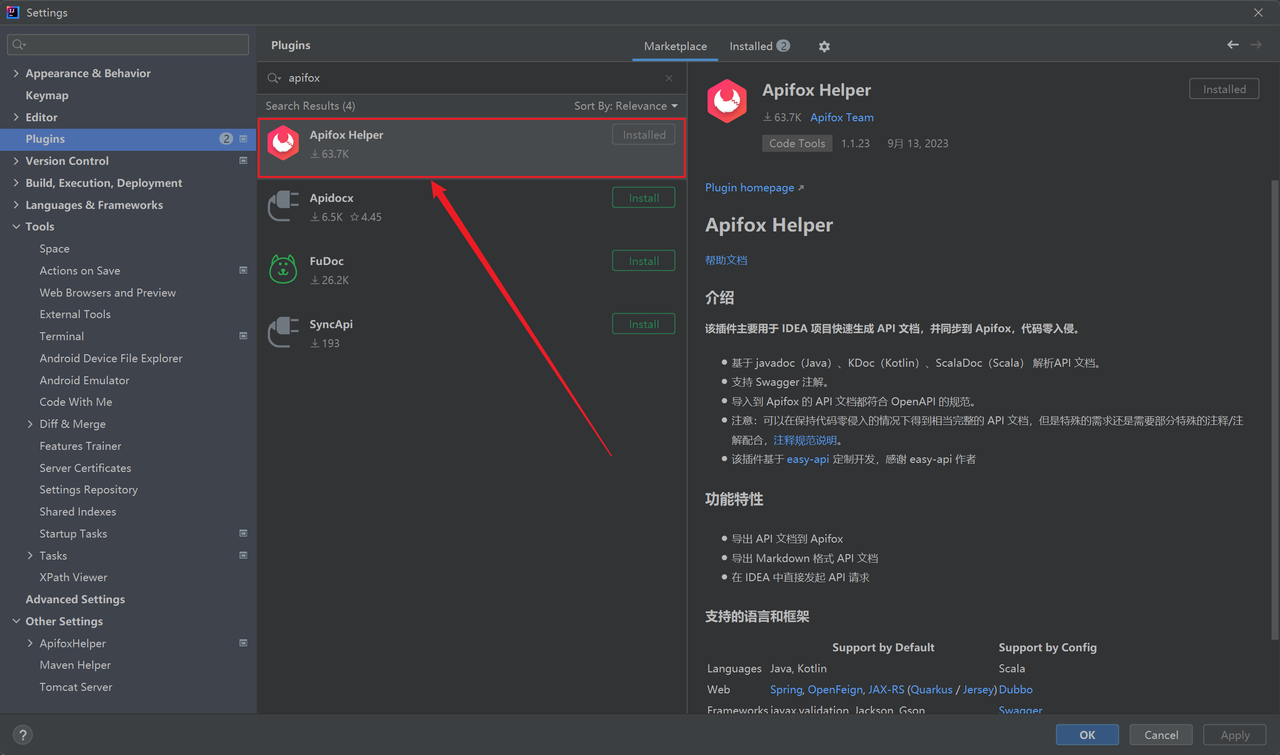
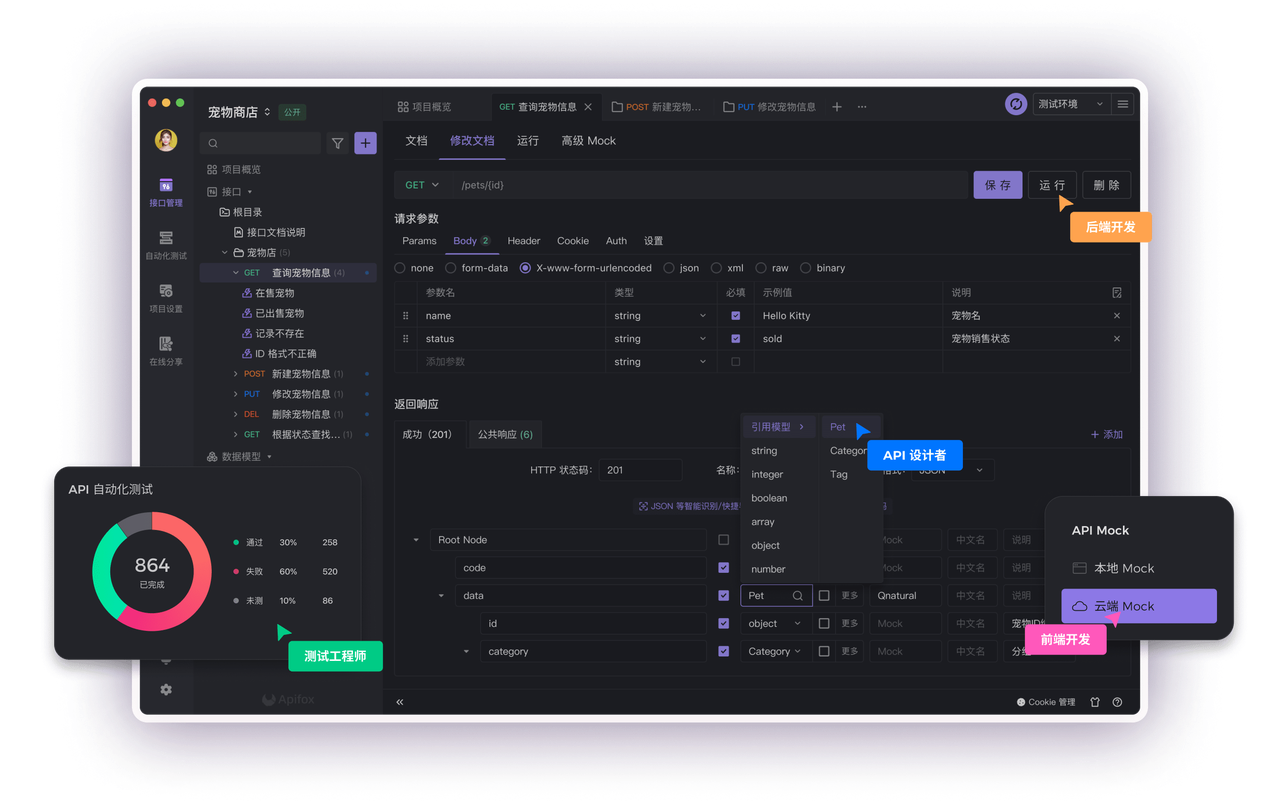
注意事项
在使用 Bean 加载功能时,需要注意以下事项:
- 确保正确配置 Bean 的作用域,以避免意外的共享状态。
- 小心避免循环依赖,这可能导致应用程序启动失败。
- 需要了解 Spring Boot 的版本兼容性,确保使用的依赖项和 Spring Boot 版本兼容。
知识扩展:
参考链接:
- Spring Boot 官方文档:https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/