GraphQL
GraphQL 是用于查询 API 的一款工具,它可以返回你想要的数据。
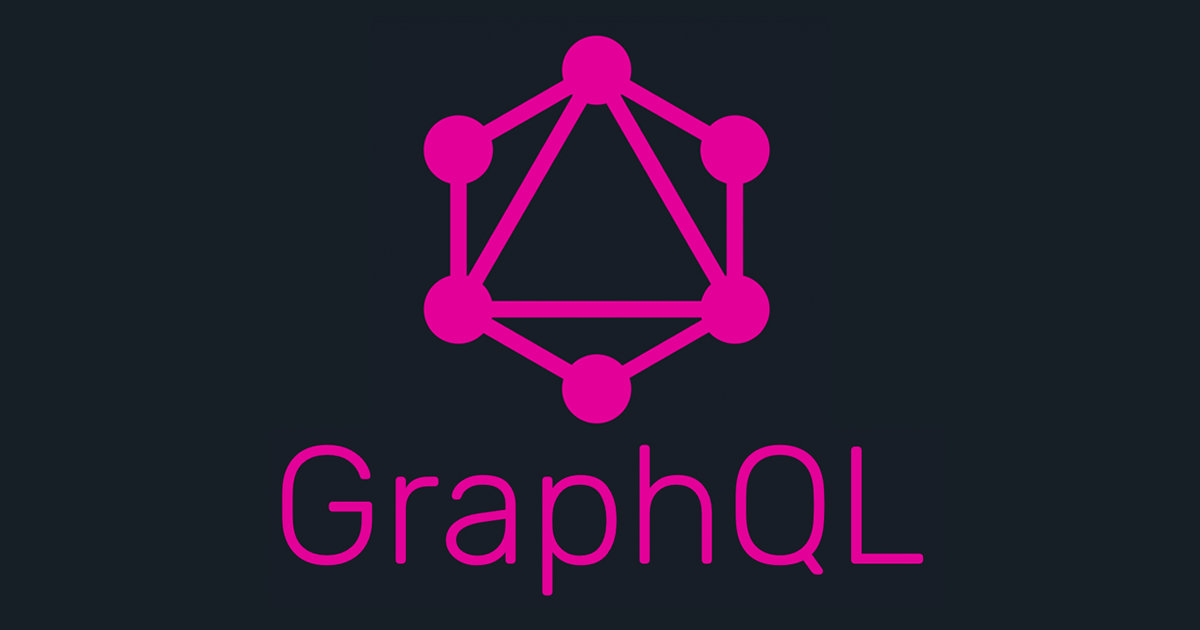
比如我们请求一个获取用户信息的接口,会返回这样的数据:
{
data: {
username: 'Sunshine',
userId: '123',
gender: 1,
age: 18,
email: 'xxxxxxx'
}
}
但其实我们并不需要这么多数据,我们只需要 username、email:
{
data: {
username: 'Sunshine',
email: 'xxxxxxx'
}
}
对于这样的需求,我们就可以使用 GraphQL。
在 Vue 中使用 GraphQL
npm 安装 GraphQL 插件
我们需要先在项目中,通过 npm 安装一些涉及到 GraphQL 的插件。
npm install --save vue-apollo graphql apollo-boost graphql-tag
创建 GraphQL 实例
我们需要创建一个 graphql.js 的文件,用来存放 GraphQL 实例。
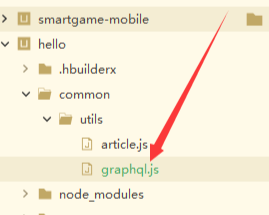
并写入以下代码:
import ApolloClient from 'apollo-boost';
const apolloClient = new ApolloClient({
uri: 'http://127.0.0.1:7001/graphql'
})
export default apolloClient;
vue.config.js 配置 GraphQL
如果你遇到以下报错的话,需要在 vue.config.js 中配置。

//vue.config.js
module.exports = {
chainWebpack: (config) => {
// GraphQL Loader
config.module // optional
.rule('graphql')
.test(/.graphql$/)
.use('graphql-tag/loader')
.loader('graphql-tag/loader')
.end()
config.module
.rule('mjs')
.test(/.mjs$/)
.include.add(/node_modules/)
.end()
.type('javascript/auto')
.end()
config.resolve.extensions
.add('.mjs')
.add('.gql')
.add('.graphql')
.end()
}
}
编写 GraphQL 请求
进入到 article.js 中写请求代码。
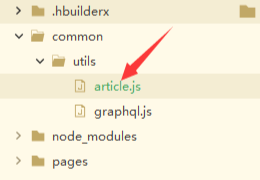
//article.js
import apolloClient from './graphql'
import gql from 'graphql-tag'
//query方式的请求
export function questionById(params) {
return apolloClient.query({
query: gql`query ($id: ID) {
questionById(id: $id) {
id
title
content
userId
}
}`,
variables: params
})
}
export function add(params) {
return apolloClient.mutate({
mutation: gql`mutation($question: addUserInput!) {
add(question:$question)
}`,
variables: params
})
}
发起 GraphQL 请求
在需要发起 GraphQL 请求的页面中,引入请求函数:
import { questionById, add } from '@/common/utils/article';
export default {
data() {
return {
list: []
}
},
created() {
this.initData()
},
methods: {
initData() {
questionById({id: 126628556787679232})
.then(res=>{
this.list = [res.data.questionById]
})
.catch(err=>{
console.log(err)
})
},
add() {
add({question: {title: 'title', content: 'content', userId: 1}}).then(res => {
if(res.data.add) {
uni.showToast({title: 'success'})
}
})
}
}
}
</script>
我们可以看到请求的 query 以及 variables。
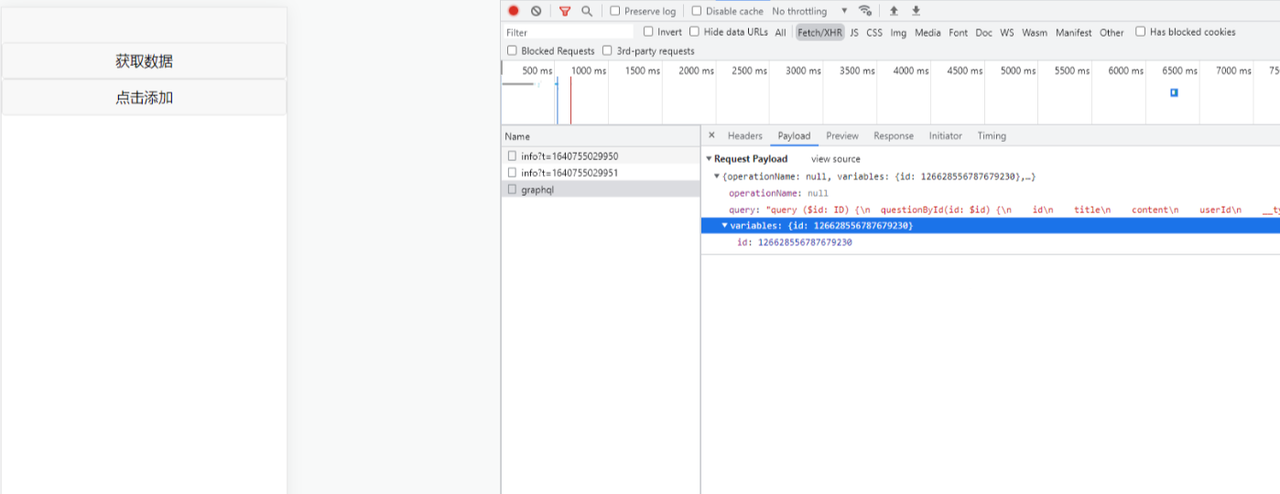
可以看到返回的数据,说明成功了!
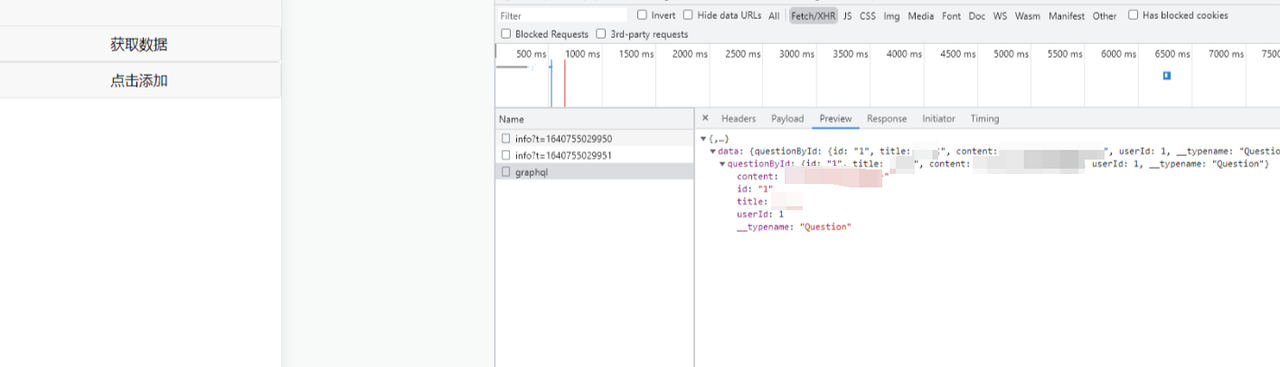
Apifox 调试 GraphQL 接口
刚刚是在 Vue 中去进行 GraphQL 请求,但是不可能一直在项目中调试,所以我们需要用到 API 工具进行调试 GraphQL 接口。
今天我们使用一款非常好用的 API 工具 Apifox 来调试 GraphQL 接口。
创建 GraphQL 接口
我们需要创建一个 GraphQL 来用于调试,填入 URL、Method、接口名称,然后点击 保存,即可创建一个接口。
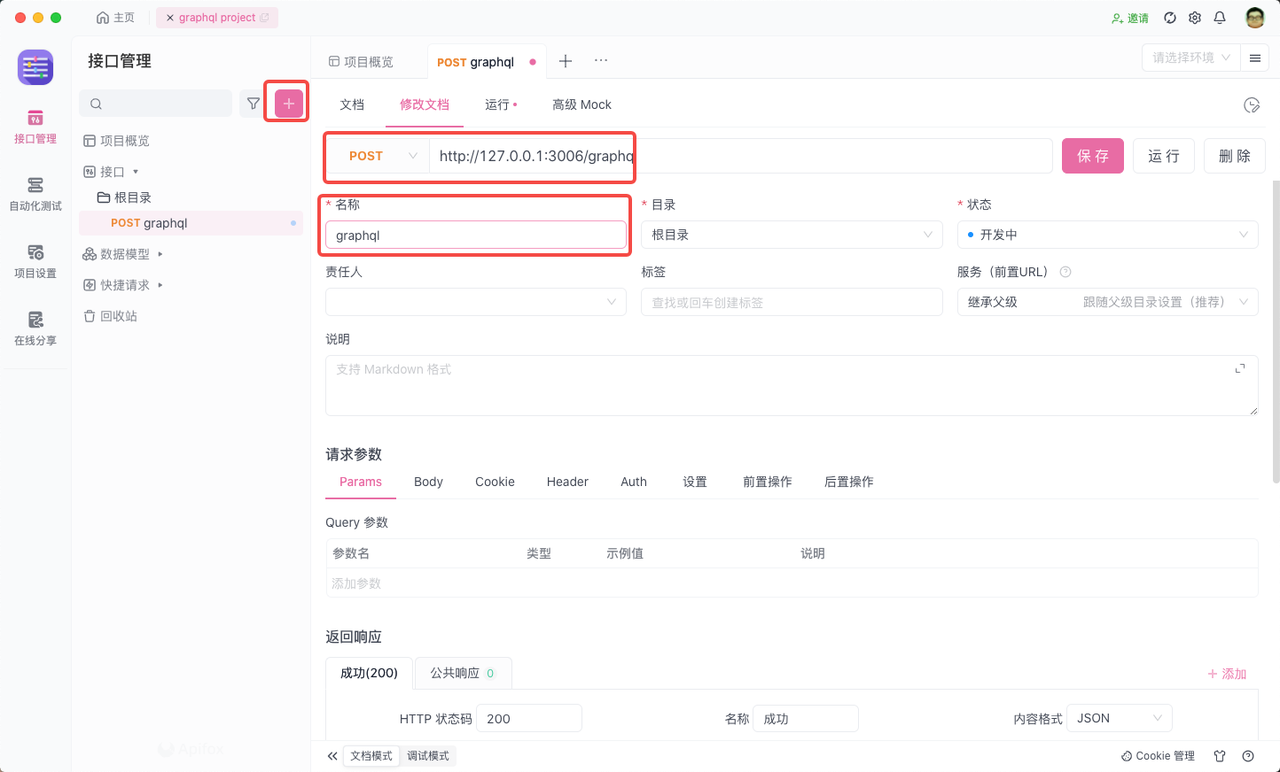
填写 query 和 variables
发送 GraphQL 请求,需要 query 和 variables,我们跳到 运行页,并填入相关参数。
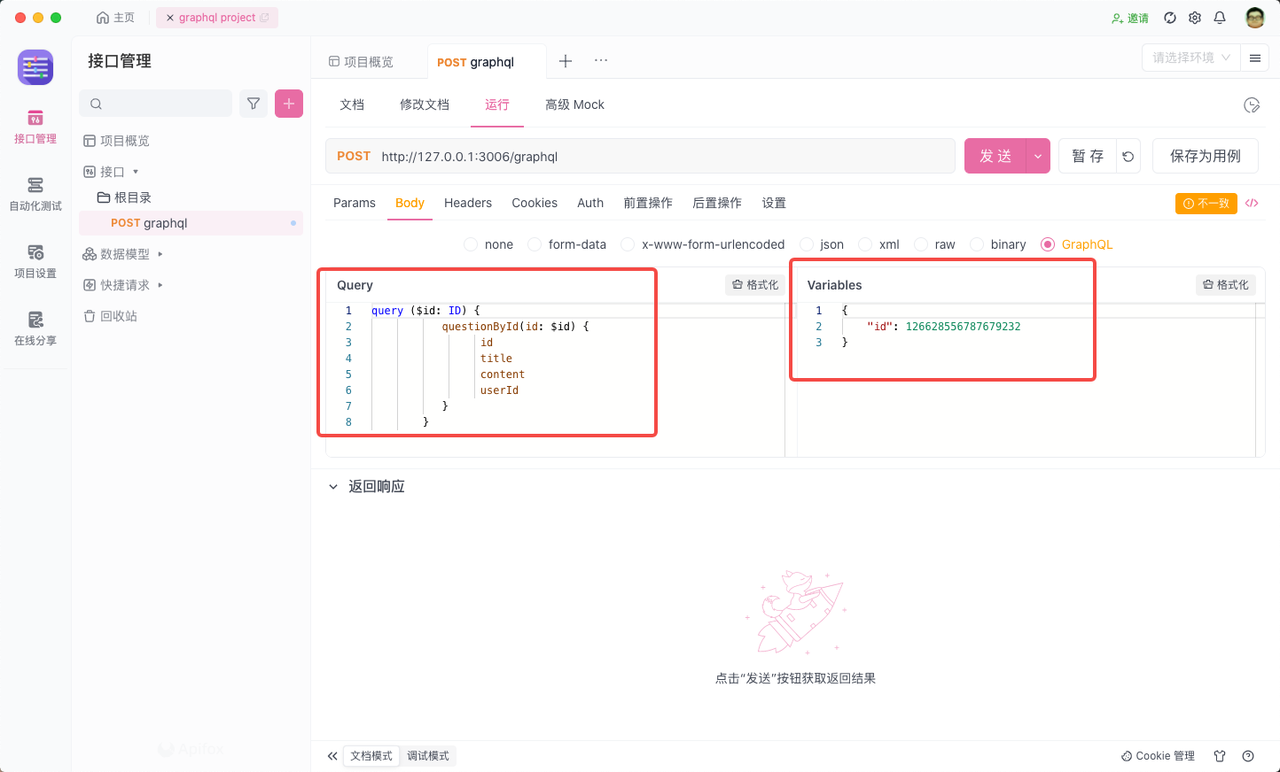
发送请求 获得响应
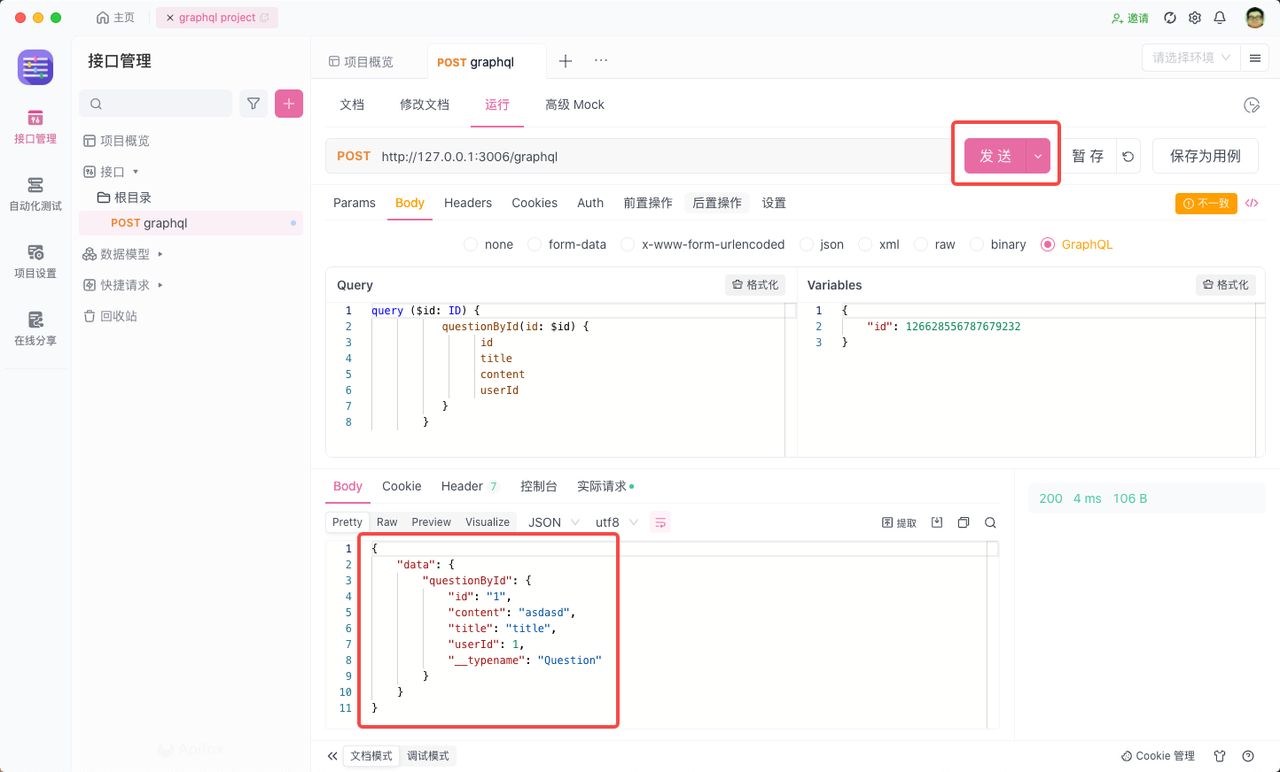
Apifox
- 集成了API 文档、API 调试、API Mock、API 自动化测试 API 一体化协作平台
- 拥有更先进的 API 设计/开发/测试工具
- Apifox = Postman + Swagger + Mock + JMeter
欢迎体验一下,完全免费的哦:在线使用 Apifox
知识扩展:
了解更多 GraphQL 相关使用技巧。