Bearer token 验证是一种常用的身份验证方式,用于保护 API 的访问权限。在 Swagger 中添加 Bearer token 可以增加 API 安全性,并限制只有具有有效访问令牌的用户才能访问受保护的资源。
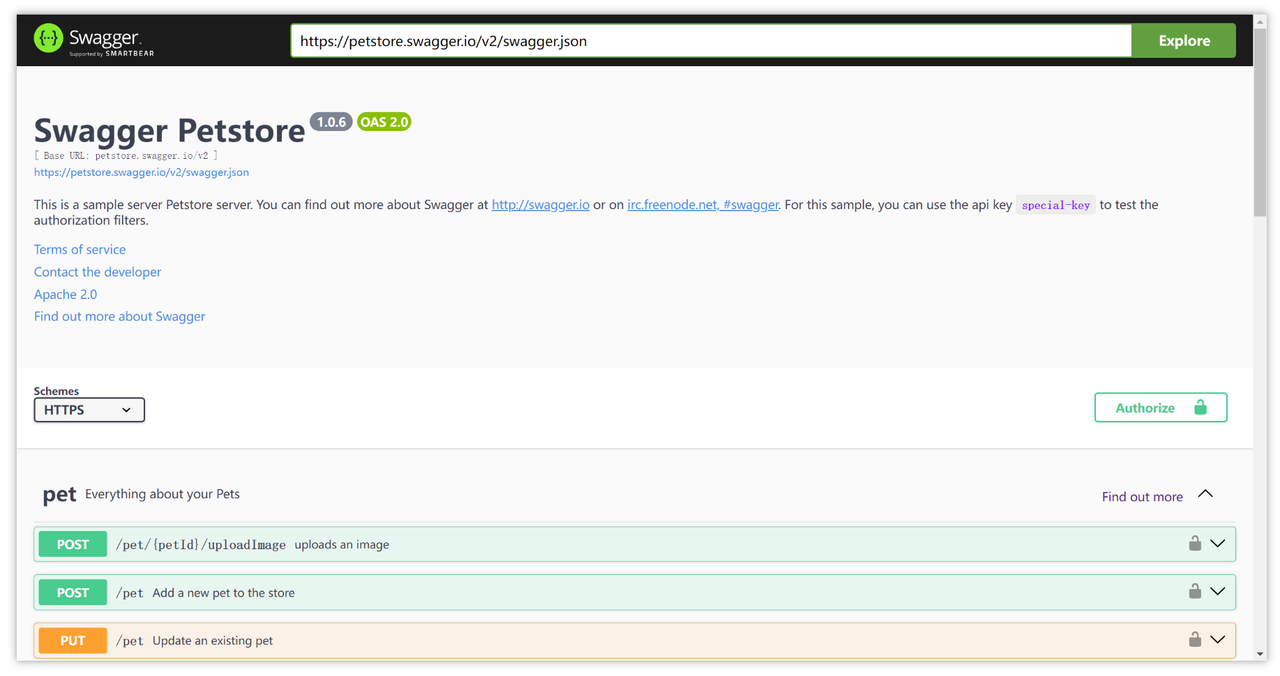
Bearer token 使用场景
在以下情况下使用 Swagger 中的 Bearer token 身份验证是有意义的:
- 在具有安全需求的项目中,例如需要限制只有认证用户才可以访问的 API。
- 在需要提供给第三方开发者的 API 文档中,以便他们了解如何在调用 API 时进行身份验证。
- 在进行 API 测试和调试时,以确保只有授权用户能够成功访问。
Bearer token 用法介绍
要在 Swagger 中添加 Bearer token,可以按照以下步骤进行:
- 确保你的 Swagger 规范(OpenAPI 规范)是正确的,并且已包含所有需要保护的路径和操作。
- 在 Swagger 规范中,为需要进行身份验证的路径和操作添加
security
定义,指定使用 Bearer token 方式进行验证。 - 在 Swagger UI 中运行时,可以在请求头部添加
Authorization
字段,并以 Bearer token 的方式携带有效的访问令牌。
实践案例
以下是一个简单的实践案例,用于在 Swagger Editor 中演示如何添加 Bearer token:
首先,在 Swagger Editor 中打开一个 YAML 文件,然后添加以下代码段:
swagger: "2.0"
info:
title: Your API
version: 1.0.0
securityDefinitions:
Bearer:
type: apiKey
name: Authorization
in: header
security:
- Bearer: []
paths:
/example:
get:
security:
- Bearer: []
responses:
200:
description: OK
这段代码中,我们首先定义了一个名为 Bearer 的安全定义,它是一个 API Key 类型的安全定义。我们将该 API Key 命名为 Authorization
并放置在请求头中。
然后,我们使用 security
关键字将安全定义应用到我们的路径上,这样在访问路径 /example
时需要提供 Bearer token。
最后,我们定义了一个简单的 GET 请求并指定了使用 Bearer token 运行。
在 Swagger Editor 中运行这段代码后,在请求 /example
时你将看到一个 "Authorization" 请求头,并且你需要在其中提供 Bearer token。
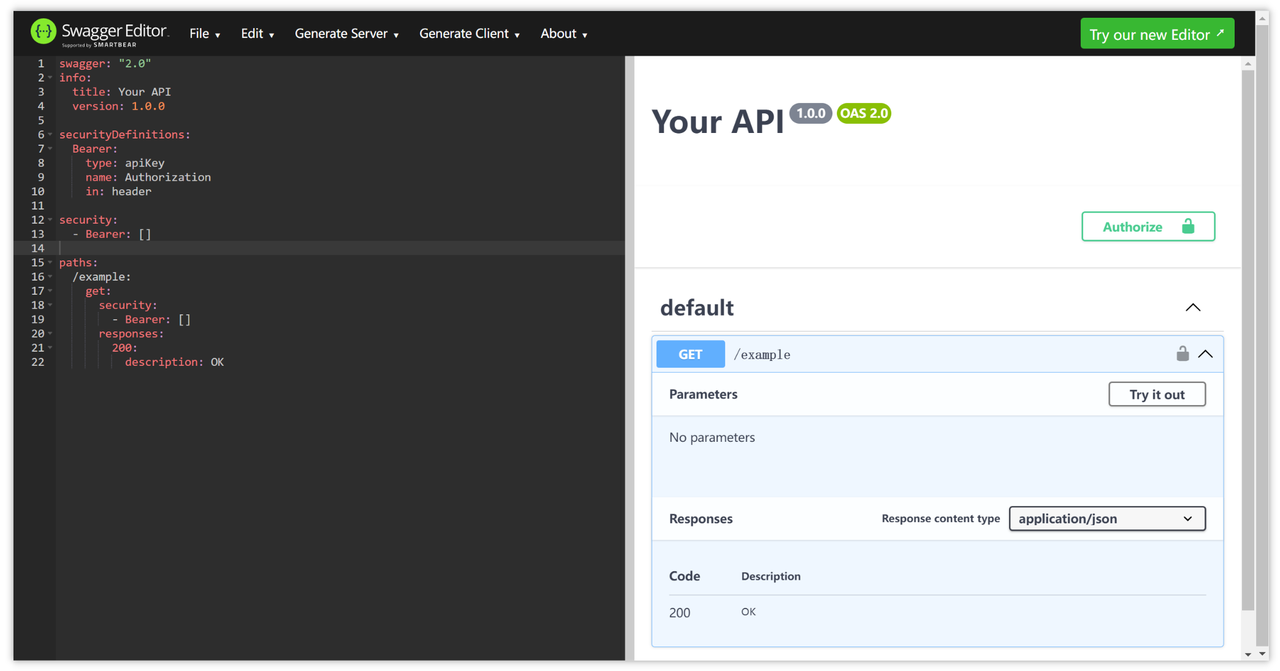
在 Spring Boot 项目中配置
为了在 Spring Boot 项目中配合 Swagger 使用 Bearer token 验证,你可以使用 Spring Security 来处理身份验证和授权的功能。具体配置步骤如下:
在 Spring Boot 项目中使用 Bearer token 进行身份验证,可以按照以下步骤操作:
- 引入相关依赖:在项目的 pom.xml 文件中,添加 Spring Security 和 JWT Token 的依赖。示例:
<dependencies>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- JWT Token -->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
</dependencies>
2. 配置 Spring Security:在项目的配置类中,配置 Spring Security 相关的安全规则和认证方式。示例:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// 配置允许访问的URL路径
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/public").permitAll()
.anyRequest().authenticated();
}
// 配置使用Bearer token进行认证
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(jwtAuthenticationProvider());
}
@Bean
public JwtAuthenticationFilter jwtAuthenticationFilter() throws Exception {
return new JwtAuthenticationFilter();
}
@Bean
public JwtAuthenticationProvider jwtAuthenticationProvider() {
return new JwtAuthenticationProvider();
}
// 配置PasswordEncoder等其他相关配置
// ...
}
3. 实现 JwtAuthenticationFilter:创建一个自定义的 JwtAuthenticationFilter,用于解析 JWT Token 并进行身份验证。示例:
public class JwtAuthenticationFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
String token = extractTokenFromRequest(request);
if (token != null) {
try {
if (isValidToken(token)) {
Authentication authentication = getAuthentication(token);
SecurityContextHolder.getContext().setAuthentication(authentication);
}
} catch (Exception e) {
// 处理验证失败的情况
}
}
filterChain.doFilter(request, response);
}
private String extractTokenFromRequest(HttpServletRequest request) {
// 从请求头部或其他位置提取Bearer token
// ...
}
private boolean isValidToken(String token) {
// 验证Bearer token是否有效
// ...
}
private Authentication getAuthentication(String token) {
// 根据Bearer token获取认证信息,并返回Authentication对象
// ...
}
}
4. 实现 JwtAuthenticationProvider:创建一个自定义的 JwtAuthenticationProvider,用于验证 JWT Token 的有效性。示例:
public class JwtAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
// 验证JWT Token的有效性
// ...
}
@Override
public boolean supports(Class<?> authentication) {
return JwtAuthenticationToken.class.isAssignableFrom(authentication);
}
}
上述示例中,JwtAuthenticationFilter 和 JwtAuthenticationProvider 是基于 JWT Token 的身份验证的自定义实现,可以根据具体的业务逻辑和需求进行进一步的定制和调整。
注意事项
在使用 Swagger 中的 Bearer token 验证功能时,可能会遇到以下常见问题:
- 配置错误导致身份验证无法生效。请确保你的 Swagger 规范和 Spring Security 配置的对应关系正确。
- 有效期管理。在使用 Bearer token 进行身份验证时,需要考虑令牌的有效期管理,以避免过期导致的访问问题。
- 安全性风险。使用 Bearer token 进行身份验证时,需要确保合理的安全策略,比如使用 HTTPS 来保护令牌的传输。
Swagger 和 Apifox 整合
Swagger 管理接口有时很不方便,缺乏一定的安全性和团队间的分享协作,所以我更推荐使用 Apifox 的 IDEA 插件。你可以在 IDEA 中自动同步 Swagger 注解到 Apifox,一键生成接口文档,多端同步,非常方便测试和维护,这样就可以迅速分享 API 给其他小伙伴。
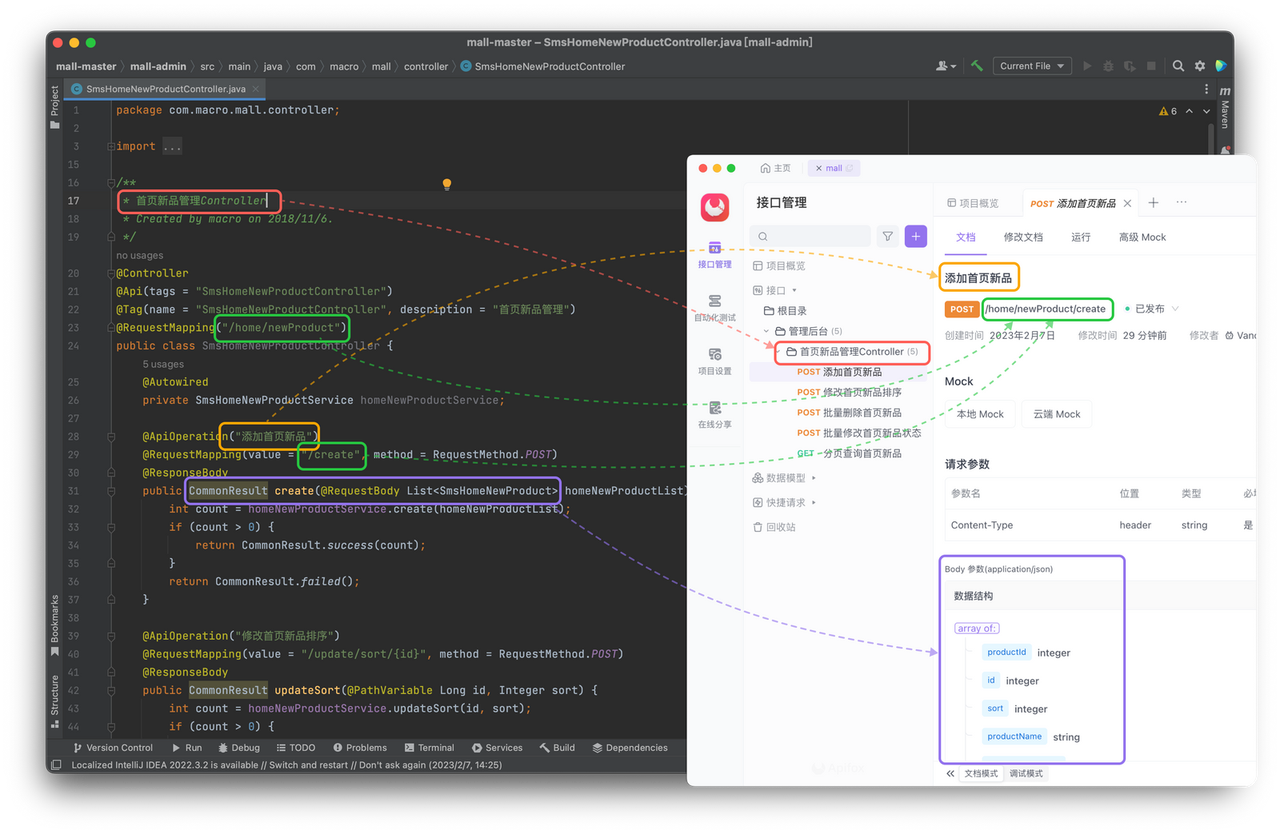
Apifox 是一个比 Postman 更强大的接口测试工具,Apifox = Postman + Swagger + Mock + JMeter,Apifox 支持调试 http(s)、WebSocket、Socket、gRPC、Dubbo 等协议的接口,并且集成了 IDEA 插件。
Apifox 的 IDEA 插件可以自动解析代码注释,并基于 Javadoc、KDoc 和 ScalaDoc 生成 API 文档。通过 IntelliJ IDEA 的 Apifox Helper 插件,开发人员可以在不切换工具的情况下将他们的文档与 Apifox 项目同步。
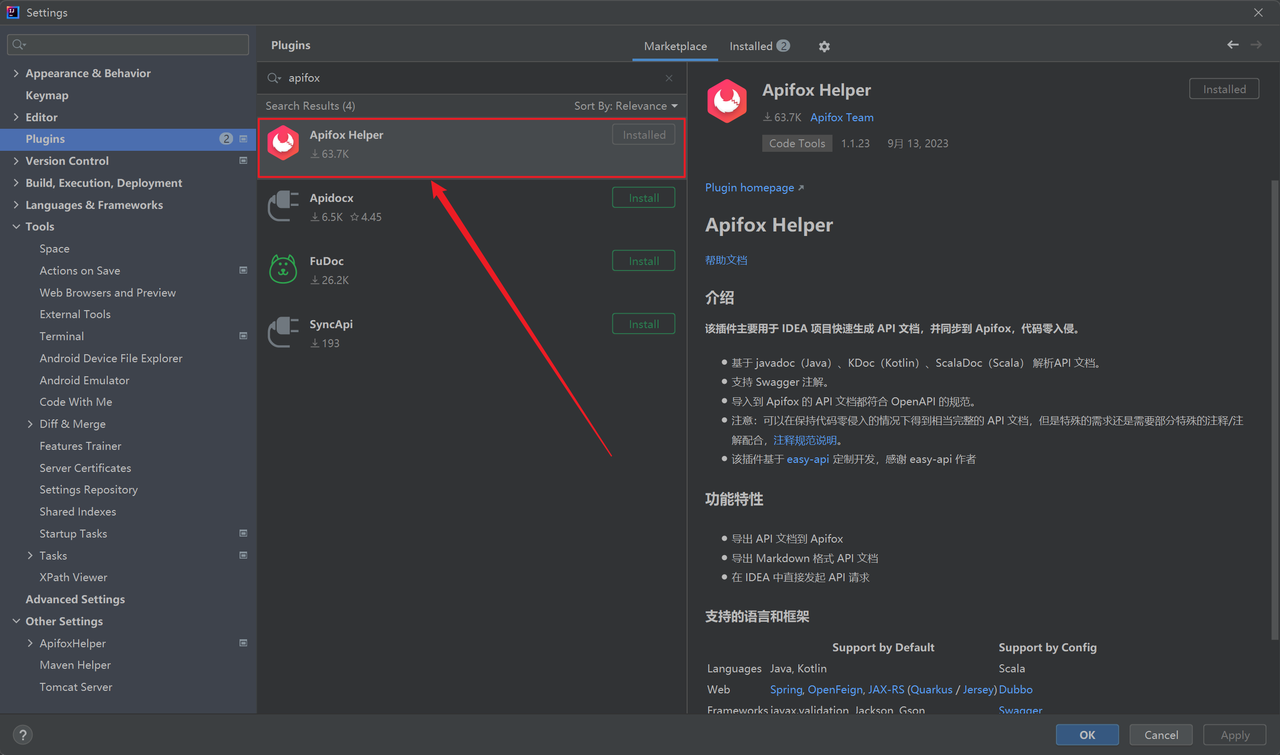
当在 IDEA 项目中有接口信息变动,只需右键点击「 Upload to Apifox」一键即可完成同步,无需奔走相告。团队成员可在 Apifox 中看到同步后的最新内容。
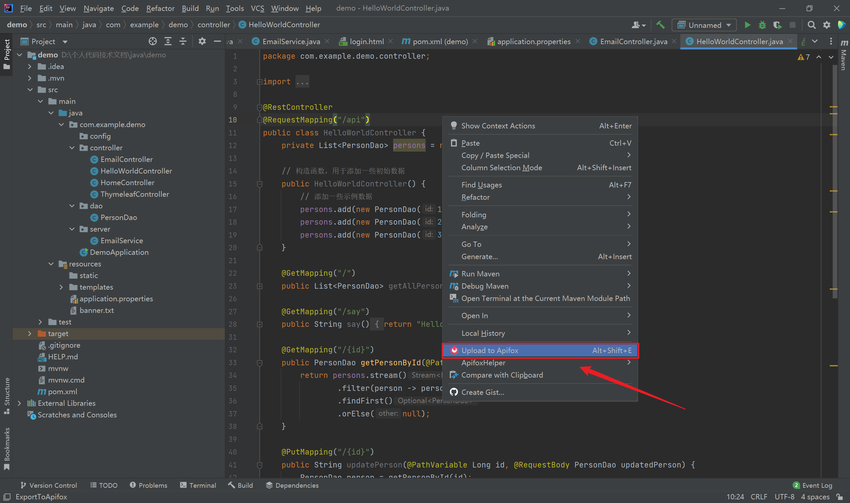
现在出发去 JetBrains 插件市场 下载试试吧!或直接在 IDEA 内 「plugin」入口直接搜索 Apifox Helper。
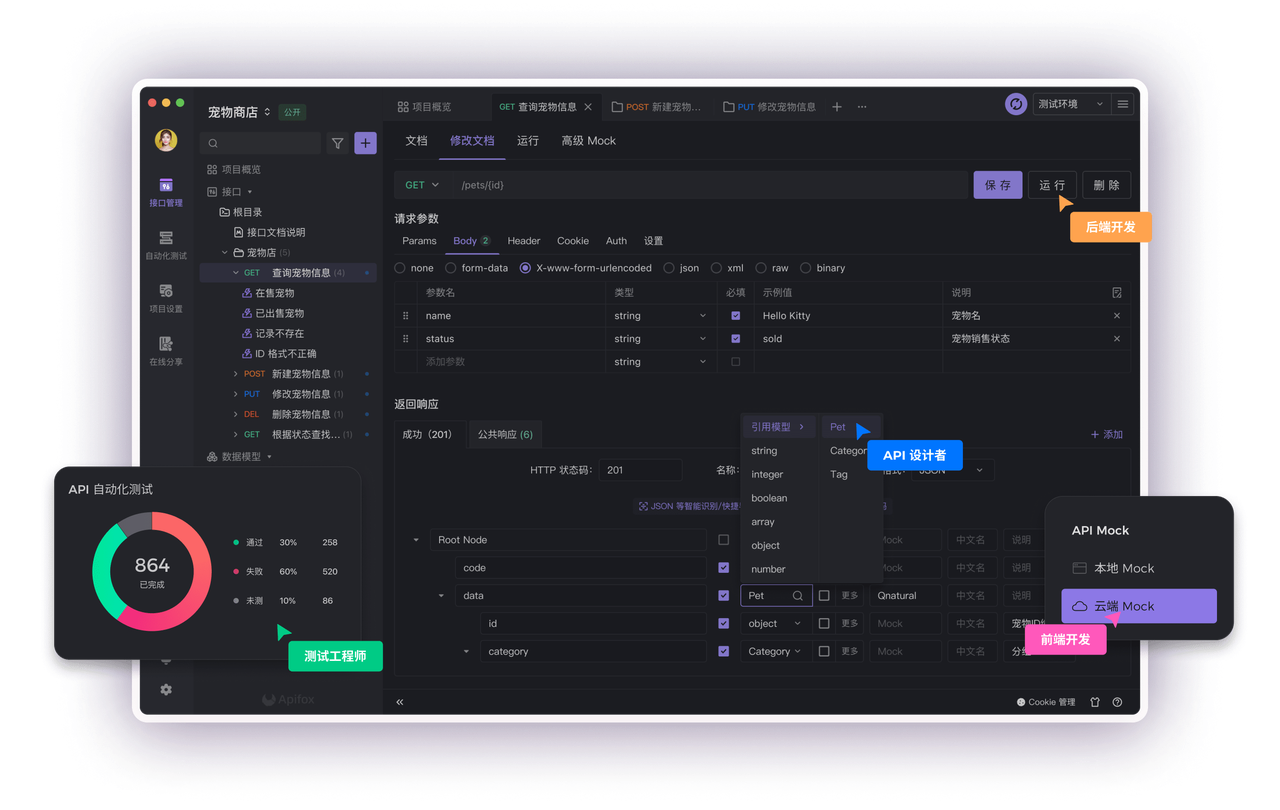
知识扩展:
参考链接:
- Swagger 官方文档:https://swagger.io/
- Spring Security 官方文档:https://spring.io/projects/spring-security