Spring Boot 中有许多常用的注解,这些注解用于配置、管理和定义 Spring Boot 应用程序的各个方面。以下是这些注解按大类和小类的方式分类,并附有解释和示例。
一、Spring Boot 核心注解
@SpringBootApplication
解释:这是一个组合注解,通常用于主应用程序类,标志着这是 Spring Boot 应用程序的入口点。它包含了其他注解,如@Configuration、@ComponentScan 和@EnableAutoConfiguration。
示例:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
@Configuration
- 解释:标志着一个类作为配置类,它通常用于定义 Bean。
- 示例:
@Configuration
public class MyConfig {
@Beanpublic MyBean myBean() {
return new MyBean();
}
}
@ComponentScan
解释:用于指定 Spring 容器扫描组件的基本包路径。
示例:
@SpringBootApplication
@ComponentScan(basePackages = "com.example")
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
二、Spring Boot Web 注解
@Controller
- 解释:标志着一个类是 Spring MVC 控制器,处理 HTTP 请求。
- 示例:
@Controller
public class MyController {
// Controller methods here
}
@RestController
解释:结合@Controller 和@ResponseBody,用于创建 RESTful 风格的控制器。 示例:
@RestController
public class MyRestController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
@RequestMapping
解释:用于映射 HTTP 请求到控制器方法,并指定 URL 路径。
示例:
@Controller
@RequestMapping("/my")
public class MyController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
三、Spring Boot Bean 注解
@Component
解释:标志着一个类是 Spring 的组件,会被 Spring 扫描并注册为一个 Bean。
示例:
@Component
public class MyComponent {
// Component logic here
}
@Service
解释:
用于标记一个类作为业务逻辑的服务组件。
示例:
@Service
public class MyService {
// Service logic here
}
@Repository
解释:
用于标记一个类作为数据访问组件,通常用于持久层。
示例:
@Repository
public class MyRepository {
// Repository logic here
}
@Controller
解释:用于标记一个类作为 Spring MVC 控制器。
示例:
@Controller
public class MyController {
// Controller logic here
}
@RestController
解释:结合@Controller 和@ResponseBody,用于创建 RESTful 风格的控制器。
示例:
@RestController
public class MyRestController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
@Configuration
解释:标志着一个类是 Spring 的配置类,通常用于定义 Bean。
示例:
@Configuration
public class MyConfig {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
这些注解用于定义和管理 Spring Bean,是 Spring Boot 应用程序中的重要组成部分。每个注解都有不同的用途和上下文,你可以根据应用程序的需求使用适当的注解。在 Spring Boot 应用程序中,使用这些注解可以轻松创建和管理 Bean,而无需显式的 XML 配置。
四、Spring Boot 数据访问注解
@Repository
解释:标志着一个类是 Spring Data 仓库,用于数据库访问。
示例:
@Repository
public class UserRepository {
// Data access methods here
}
@Entity
解释:用于定义 JPA 实体类,映射到数据库表。
示例:
@Entity
public class User {
// Entity fields and methods here
}
五、Spring Boot 依赖注入注解
@Autowired
解释:用于自动装配 Bean,通常与构造函数、Setter 方法或字段一起使用。
示例:
@Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
}
@Qualifier
解释:与@Autowired 一起使用,用于指定要注入的 Bean 的名称。
示例:
@Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(@Qualifier("myRepository") MyRepository repository) {
this.repository = repository;
}
}
六、其他常用注解
@Value
- 解释:用于注入属性值,通常从配置文件中获取。
- 示例:
@Component
public class MyComponent {
@Value("${my.property}")
private String myProperty;
}
@ConfigurationProperties
解释:用于将配置属性绑定到一个 POJO 类,通常用于从配置文件中读取属性值。 示例:
@Configuration
@ConfigurationProperties(prefix = "my")
public class MyProperties {
private String property1;
private int property2;
// Getters and setters
}
这只是 Spring Boot 中一些常用注解的分类和示例,还有许多其他注解可用于更专业的用例。根据你的需求,你可以选择使用适当的注解来配置和管理你的 Spring Boot 应用程序。
使用 Apifox 测试和管理接口
如果你是 JAVA 开发者,你经常需要与 API 打交道,确保你的应用程序能够正常工作。这时,一个强大的接口测试工具就会派上用场。
Apifox 是一个比 Postman 更强大的接口测试工具,Apifox = Postman + Swagger + Mock + JMeter,Apifox 支持调试 http(s)、WebSocket、Socket、gRPC、Dubbo 等协议的接口,并且集成了 IDEA 插件。在开发完接口后,可以通过 Apifox 的 IDEA 插件一键自动生成接口文档,多端同步,非常方便测试和维护。
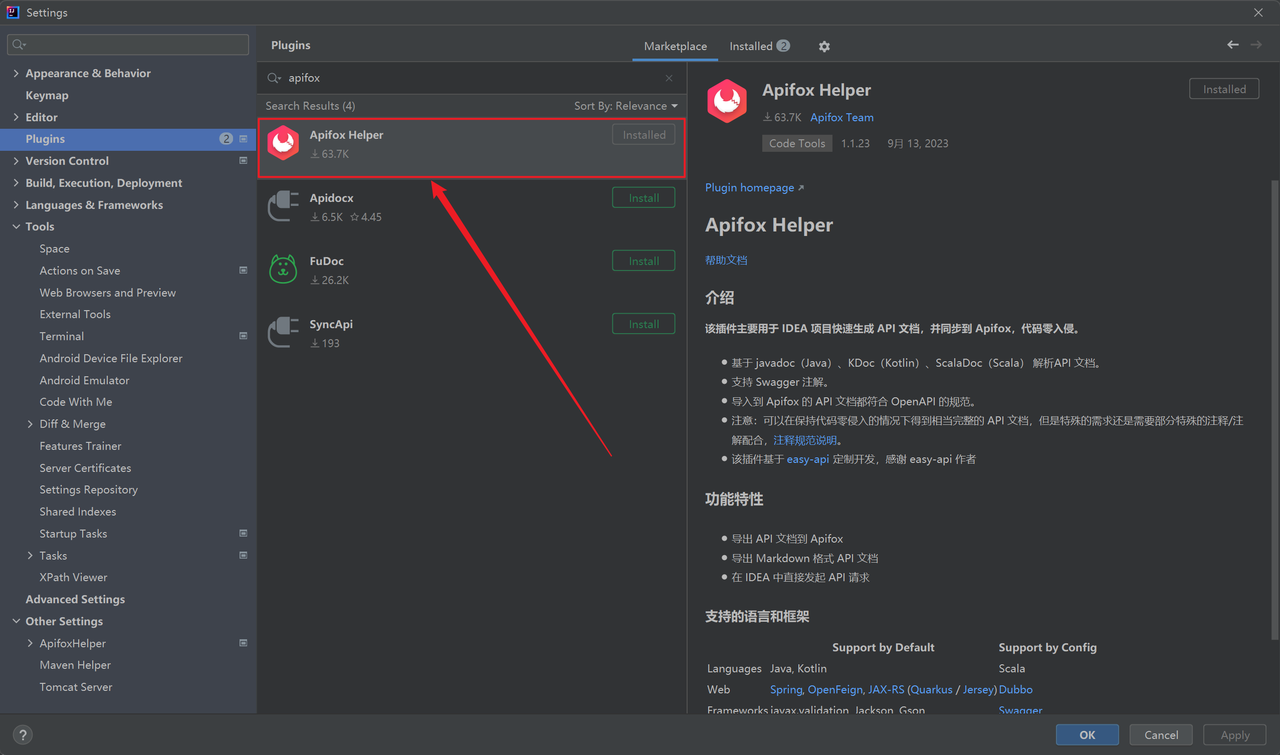
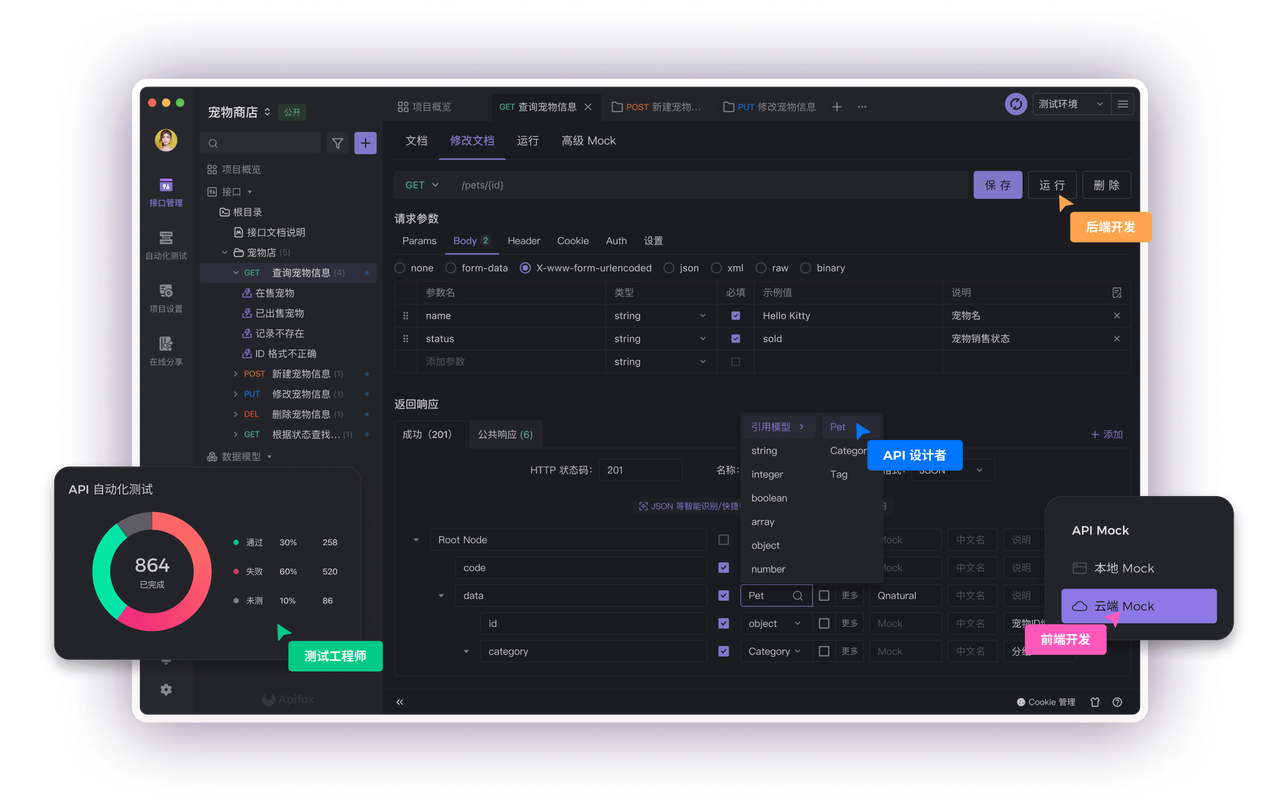
知识扩展: