@Autowired 注解是Spring框架的一部分,用于自动装配(自动注入)Spring Bean到其他Bean中。它的主要作用是帮助我们消除手动配置Bean依赖关系的繁琐工作,使代码更加简洁和可维护。通过使用 @Autowired
注解,我们可以轻松地将一个Bean注入到另一个Bean中,而无需手动创建实例或者进行繁琐的配置。
@Autowired
注解为项目提供了以下优势:
- 减少了手动配置Bean之间的依赖关系的工作,提高了开发效率。
- 降低了代码的耦合度,使得代码更容易测试和维护。
- 提供了一种声明式的方式来定义Bean之间的依赖关系,增强了代码的可读性。
@autowired 注解使用场景
在什么情况下使用 @Autowired
注解是有意义的呢?以下是一些常见的使用场景:
- 依赖注入:当一个Bean需要依赖于另一个Bean时,可以使用
@Autowired
注解来自动注入所需的Bean。 - 服务层:在服务层(Service)中,通常需要依赖于数据访问层(Repository)的Bean,可以使用
@Autowired
注解来注入数据访问层的实例。 - 控制器层:在控制器层(Controller)中,需要注入服务层的Bean,以处理请求并返回响应。
- 测试:在单元测试中,使用
@Autowired
注解可以轻松地注入所需的依赖,使测试更容易编写和维护。
@autowired 注解配置
要在Spring Boot项目中使用 @Autowired
注解,需要执行以下步骤:
1.引入Spring Boot依赖:确保你的项目已经引入了Spring Boot的依赖,可以在Maven或Gradle中配置相应的依赖项。
2.创建Bean:在项目中创建需要注入的Bean,并使用 @Autowired
注解标记需要注入的属性或构造函数。
3.启用自动装配:在Spring Boot应用程序的配置类上添加 @SpringBootApplication
注解,它会启用自动装配功能。
4.使用 @Autowired 注解:在需要注入Bean的地方使用 @Autowired
注解。Spring Boot会自动查找并注入相应的Bean。
下面是一个示例配置类的代码:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
以下是一个示例Service类,演示了如何使用 @Autowired
注解注入另一个Bean:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
// ...
}
实践案例
假设你正在构建一个简单的学生管理系统,有两个类:Student
表示学生,StudentService
表示处理学生数据的服务类。StudentService
需要依赖StudentRepository
来访问学生数据。
首先,定义Student
类:
public class Student {
private String name;
// 构造函数、getter和setter方法
}
然后,创建StudentRepository
用于访问学生数据:
@Repository
public class StudentRepository {
public Student getStudentByName(String name) {
// 在实际应用中,这里会从数据库或其他数据源中获取学生数据
Student student = new Student();
student.setName(name);
return student;
}
}
接下来,创建StudentService
类并使用@Autowired
注解来注入StudentRepository
依赖:
@Service
public class StudentService {
private final StudentRepository studentRepository;
@Autowired
public StudentService(StudentRepository studentRepository) {
this.studentRepository = studentRepository;
}
public Student findStudentByName(String name) {
return studentRepository.getStudentByName(name);
}
// 其他学生管理方法
}
在上述代码中,StudentService
的构造函数使用@Autowired
注解将StudentRepository
依赖注入。这意味着当我们创建StudentService
实例时,Spring Boot将自动查找并注入所需的StudentRepository
实例。
最后,我们可以创建一个简单的控制器类来使用StudentService
来查找学生:
@RestController
@RequestMapping("/students")
public class StudentController {
private final StudentService studentService;
@Autowired
public StudentController(StudentService studentService) {
this.studentService = studentService;
}
@GetMapping("/{name}")
public ResponseEntity<Student> getStudent(@PathVariable String name) {
Student student = studentService.findStudentByName(name);
if (student != null) {
return new ResponseEntity<>(student, HttpStatus.OK);
} else {
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
}
// 其他学生相关的API
}
在上述代码中,StudentController
的构造函数也使用@Autowired
注解,将StudentService
依赖注入。然后,我们可以使用StudentService
来查找学生。
这个简单的例子展示了如何使用@Autowired
注解来实现依赖注入,以简化Spring Boot应用程序中的组件管理和依赖解析。通过这种方式,我们可以更轻松地组织和维护应用程序的代码。
使用 Apifox 测试和管理接口
如果你是 JAVA 开发者,你经常需要与 API 打交道,确保你的应用程序能够正常工作。这时,一个强大的接口测试工具就会派上用场。
Apifox 是一个比 Postman 更强大的接口测试工具,Apifox = Postman + Swagger + Mock + JMeter,Apifox 支持调试 http(s)、WebSocket、Socket、gRPC、Dubbo 等协议的接口,并且集成了 IDEA 插件。在开发完接口后,可以通过 Apifox 的 IDEA 插件一键自动生成接口文档,多端同步,非常方便测试和维护。
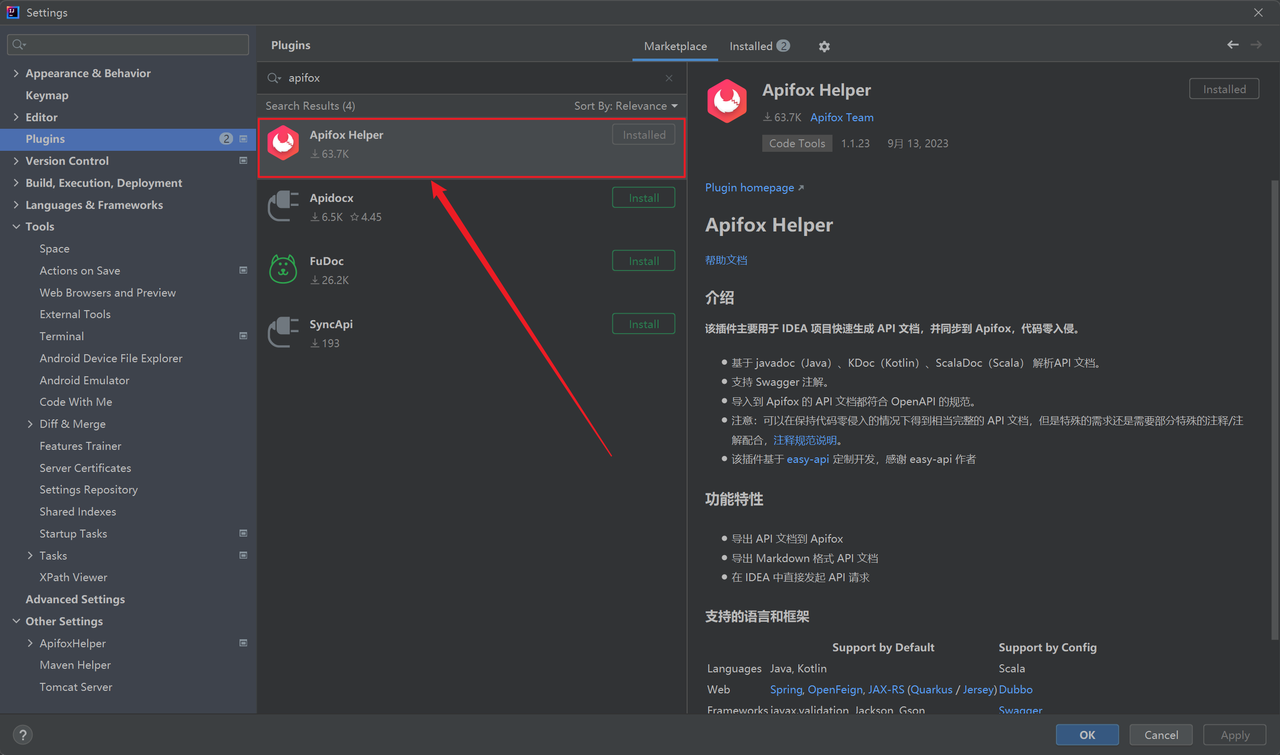
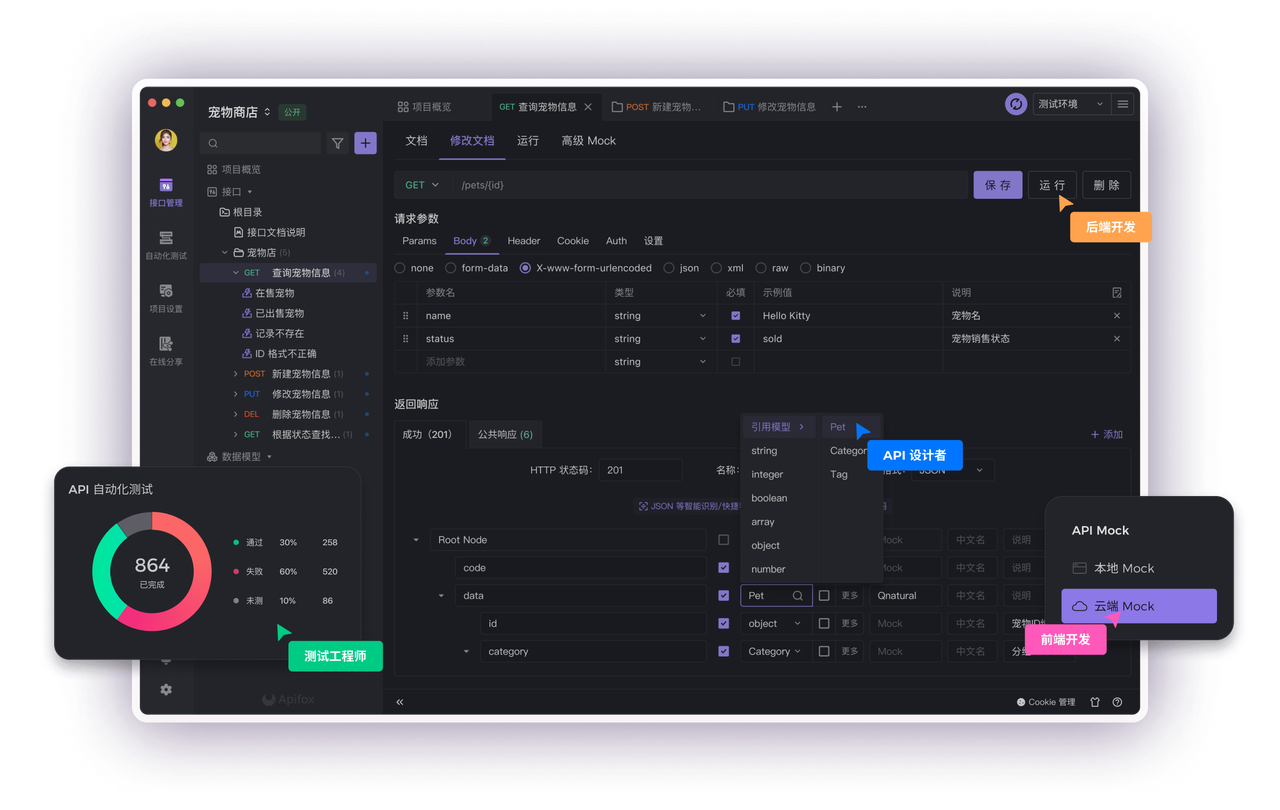
注意事项
在使用 @Autowired
注解时,需要注意以下事项:
- 确保依赖的Bean已经被Spring容器管理,即它们需要被标记为
@Component
、@Service
、@Repository
等注解之一。 - 如果有多个符合条件的Bean,Spring可能会抛出
NoUniqueBeanDefinitionException
异常。在这种情况下,你可以使用@Qualifier
注解来指定要注入的Bean。 @Autowired
注解可以用在构造函数、属性、和方法上,根据你的需求选择合适的位置。
知识扩展:
参考链接:
- Spring Framework Documentation:https://spring.io/projects/spring-framework
- Spring Boot Documentation:https://spring.io/projects/spring-boot