gRPC 是一个高性能、开源和通用的 RPC 框架,它基于 HTTP/2 协议,使用 Protobuf 作为其接口描述语言。在 Java 中使用 gRPC
,你需要先设置项目并添加所需的依赖。以下是在 Java 项目中安装和配置 gRPC
的具体步骤,最后使用调试工具对 gRPC 进行调试。
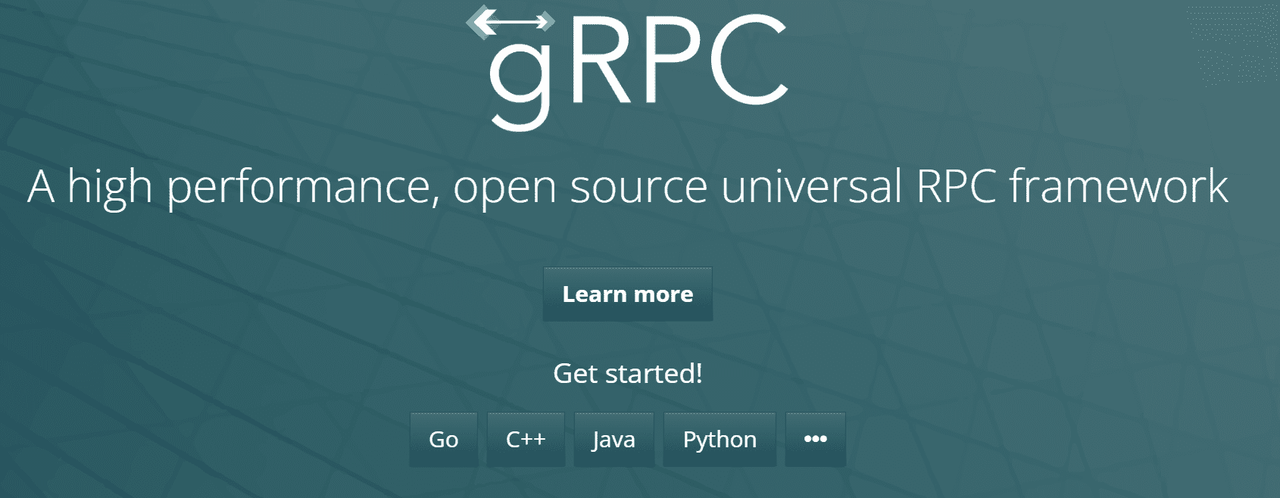
gRPC 调试工具
为了优化 gRPC 的调试体验,选择一个可靠的调试工具至关重要。这里我比较推荐使用 Apifox —— 一款支持多种协议(如 http、https、WebSocket、Socket、gRPC、Dubbo 等)的全能接口测试工具。下面,我们将通过具体步骤演示如何在 Python 中使用 gRPC,并借助 Apifox 进行高效调试。
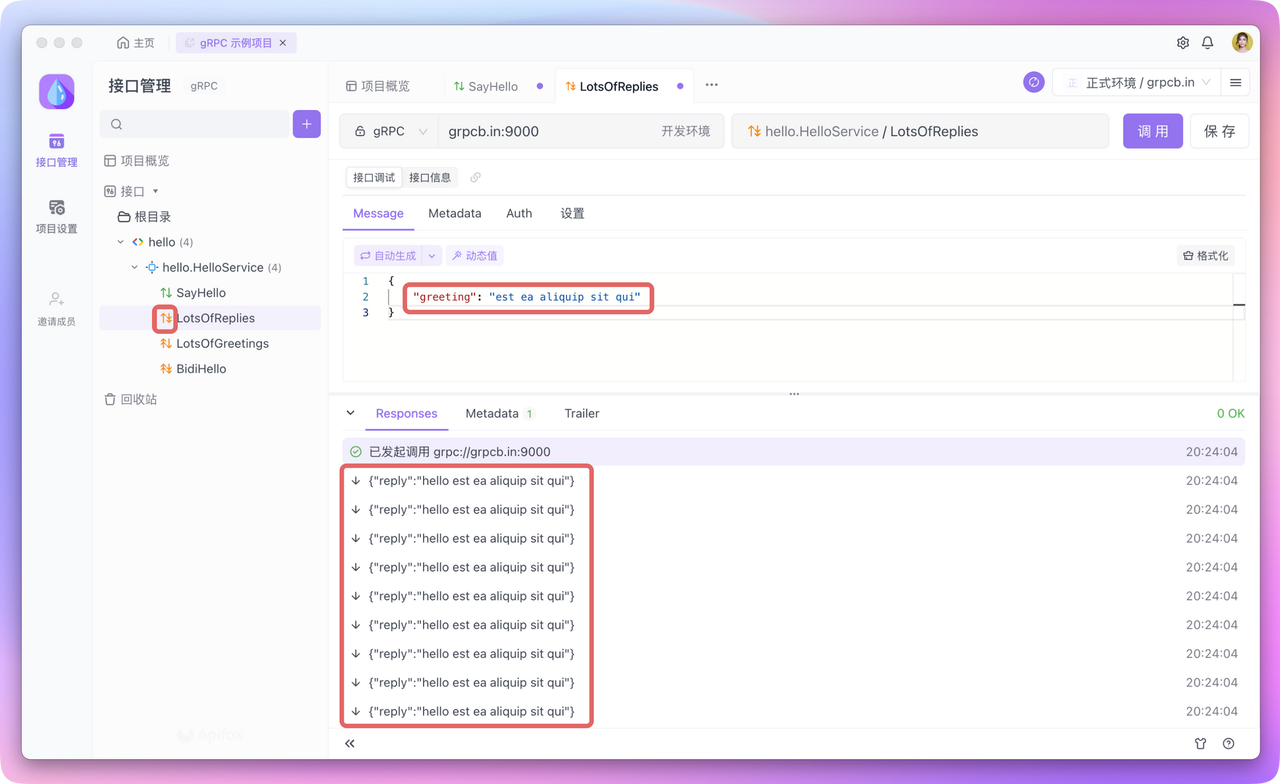
Java 实现 gRPC 具体步骤
步骤 1: 设置 Java 开发环境
确保你的开发环境中已经安装了 Java SDK。使用以下命令查看是否已安装 Java:
java -version
步骤 2: 创建一个新的 Java 项目
创建一个新的 Java 项目,可以使用命令行工具如 Maven 或 Gradle,也可以在 IDE(如 IntelliJ IDEA 或 Eclipse)中创建。
如果使用 Maven,可以创建如下的 pom.xml
文件:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>grpc-demo</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- 添加 gRPC 依赖 -->
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty</artifactId>
<version>1.47.0</version> <!-- 使用时请替换为最新版本 -->
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.47.0</version> <!-- 同理 -->
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.47.0</version> <!-- 同理 -->
</dependency>
</dependencies>
<build>
<plugins>
<!-- 添加 Protobuf 编译器插件 -->
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version> <!-- 使用时请替换为最新版本 -->
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.19.1:exe:${os.detected.classifier}</protocArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
如果使用 Gradle,可以创建如下的 build.gradle
文件:
plugins {
id 'java'
id 'com.google.protobuf' version '0.8.17'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
// 添加 gRPC 依赖
implementation 'io.grpc:grpc-netty:1.47.0' // 使用时请替换为最新版本
implementation 'io.grpc:grpc-protobuf:1.47.0' // 同理
implementation 'io.grpc:grpc-stub:1.47.0' // 同理
}
protobuf {
protoc {
artifact = 'com.google.protobuf:protoc:3.19.1'
}
plugins {
grpc {
artifact = 'io.grpc:protoc-gen-grpc-java:1.47.0' // 使用时请替换为最新版本
}
}
generateProtoTasks {
all().each { task ->
task.plugins {
grpc {}
}
}
}
}
步骤 3: 编写 Proto 文件
创建 .proto
文件定义你的服务和消息类型。例如,创建 helloworld.proto
文件:
syntax = "proto3";
package helloworld;
// 定义 Greeter 服务
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
// 请求消息
message HelloRequest {
string name = 1;
}
// 响应消息
message HelloReply {
string message = 1;
}
确保之前的步骤已经完成,我们继续往下进行。
步骤 4: 编译 Proto 文件
在项目根目录下运行 Maven 或 Gradle 构建命令,以生成相关的 Java 类。
对于 Maven 项目运行:
mvn clean compile
对于 Gradle 项目运行:
./gradlew build
构建过程将会根据你的 .proto
文件,在指定的目录(如 target/generated-sources/protobuf/java
对于 Maven 或 build/generated/source/proto/main/java
对于 Gradle)中生成对应的 Java 服务接口和消息类。
步骤 5: 实现服务
基于生成的接口实现你的服务。在这个例子中,我们需要实现Greeter
服务。
import io.grpc.stub.StreamObserver;
import helloworld.GreeterGrpc;
import helloworld.Helloworld.HelloReply;
import helloworld.Helloworld.HelloRequest;
public class GreeterServiceImpl extends GreeterGrpc.GreeterImplBase {
@Override
public void sayHello(HelloRequest req, StreamObserver<HelloReply> responseObserver) {
HelloReply reply = HelloReply.newBuilder().setMessage("Hello " + req.getName()).build();
responseObserver.onNext(reply);
responseObserver.onCompleted();
}
}
步骤 6: 运行服务
创建并启动 gRPC 服务器以便客户端能够连接和使用服务。
import io.grpc.Server;
import io.grpc.ServerBuilder;
public class HelloWorldServer {
private Server server;
private void start() throws IOException {
/* The port on which the server should run */
int port = 50051;
server = ServerBuilder.forPort(port)
.addService(new GreeterServiceImpl())
.build()
.start();
System.out.println("Server started, listening on " + port);
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
System.err.println("*** shutting down gRPC server since JVM is shutting down");
HelloWorldServer.this.stop();
System.err.println("*** server shut down");
}));
}
private void stop() {
if (server != null) {
server.shutdown();
}
}
public static void main(String[] args) throws IOException, InterruptedException {
final HelloWorldServer server = new HelloWorldServer();
server.start();
server.blockUntilShutdown();
}
}
上面的代码创建了一个 gRPC 服务器在端口 50051
上监听,并注册了我们的服务实现。在 main
方法中启动服务器。
步骤 7: 编写和运行客户端
客户端用于连接服务器并调用服务。
import io.grpc.ManagedChannel;
import io.grpc.ManagedChannelBuilder;
import helloworld.GreeterGrpc;
import helloworld.Helloworld.HelloReply;
import helloworld.Helloworld.HelloRequest;
public class HelloWorldClient {
private final GreeterGrpc.GreeterBlockingStub blockingStub;
public HelloWorldClient(String host, int port) {
ManagedChannel channel = ManagedChannelBuilder.forAddress(host, port)
.usePlaintext() // Disable SSL to avoid needing certificates.
.build();
blockingStub = GreeterGrpc.newBlockingStub(channel);
}
public void greet(String name) {
HelloRequest request = HelloRequest.newBuilder().setName(name).build();
HelloReply response;
try {
response = blockingStub.sayHello(request);
System.out.println("Greeting: " + response.getMessage());
} catch (StatusRuntimeException e) {
System.err.println("RPC failed: " + e.getStatus());
}
}
public static void main(String[] args) throws Exception {
HelloWorldClient client = new HelloWorldClient("localhost", 50051);
client.greet("world");
}
}
上面的客户端代码尝试连接到服务器并向 Greeter
服务发送一个 "world" 请求,接着打印响应。
运行服务器端程序,然后再运行客户端程序,客户端应该能够从服务器接收到 "Hello world" 的回应。
使用 Apifox 调试 gRPC
目前,市面上能够兼容 gRPC 接口的调试与管理工具相对有限,但值得注意的是,Apifox 作为业界领先的接口管理工具,已经支持 gRPC 接口的调试和管理功能,这一功能的推出使得在微服务架构中广泛应用的 gRPC 变得更加便捷。Apifox 提供全面的兼容性,涵盖 gRPC 的四种调用类型:
- Unary:一元调用
- Server Streaming:服务端流
- Client Streming:客户端流
- Bidirectional Streaming:双向流
下文将通过一个示例场景简要演示如何在 Apifox 中新建 gRPC 项目并针对接口发起调试。
步骤1:新建 gRPC 项目
在 Apifox 中登录并新建一个 gRPC 项目,点击“新建项目”按钮,选择 gRPC 类型,填写项目名称后轻点“新建”按钮。
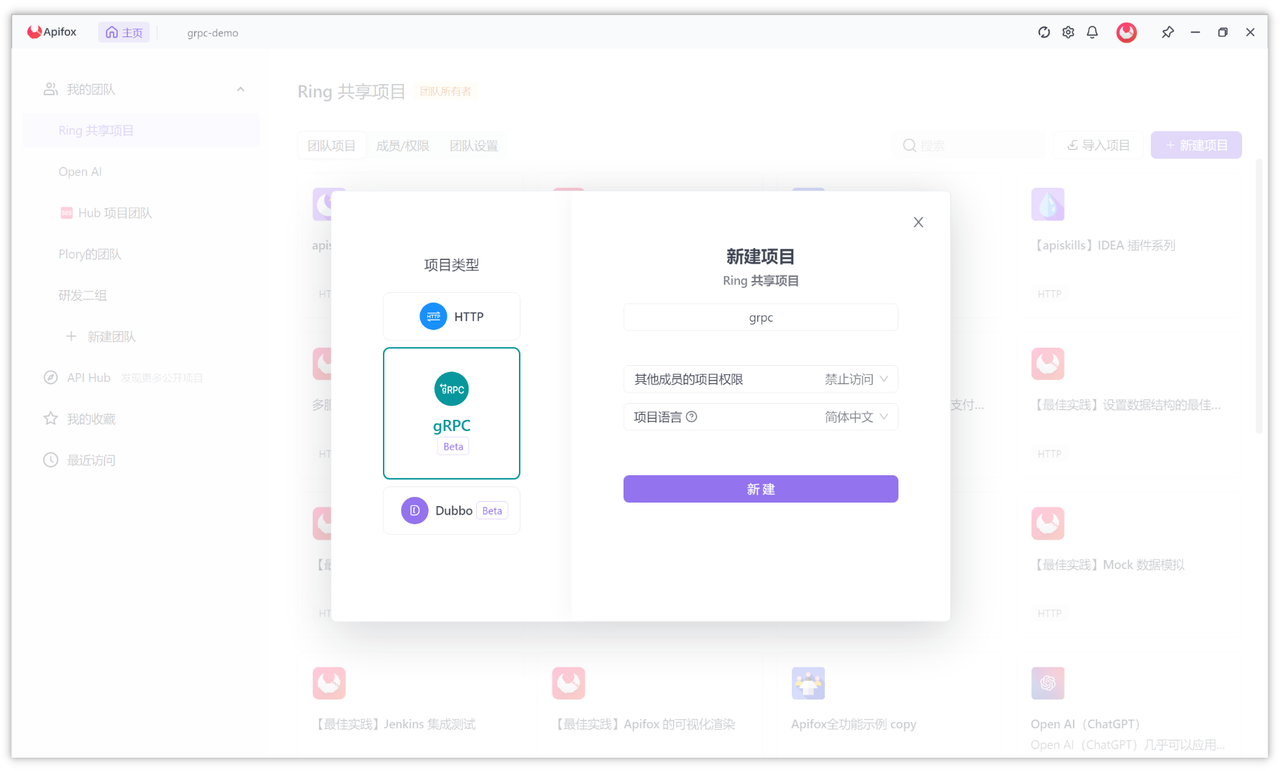
步骤2:导入.proto
文件
导入定义 gRPC 接口所使用的服务、方法和消息的 .proto
文件。你可以将文件拖拽至其中或使用文件在线 URL 完成导入。
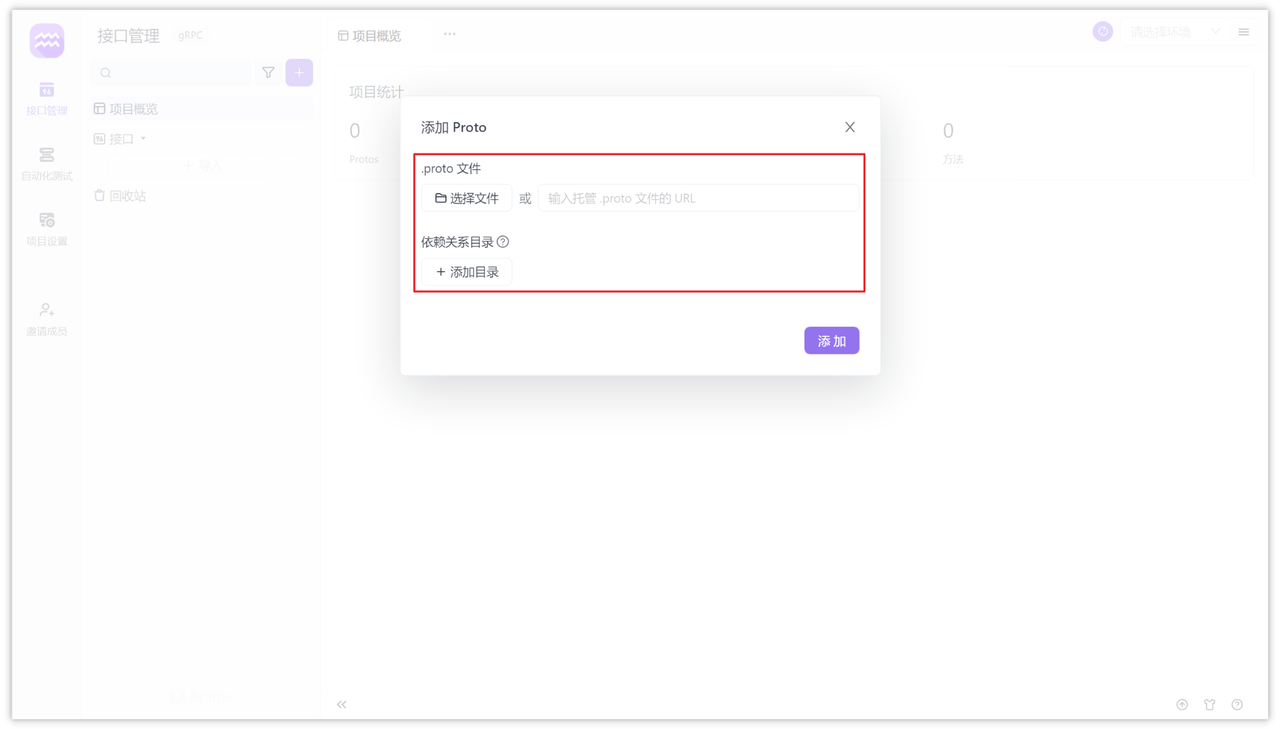
步骤3:调试 gRPC
文件导入后,Apifox 将基于 .proto
文件内容生成对应的接口信息,然后就可以进行调试。
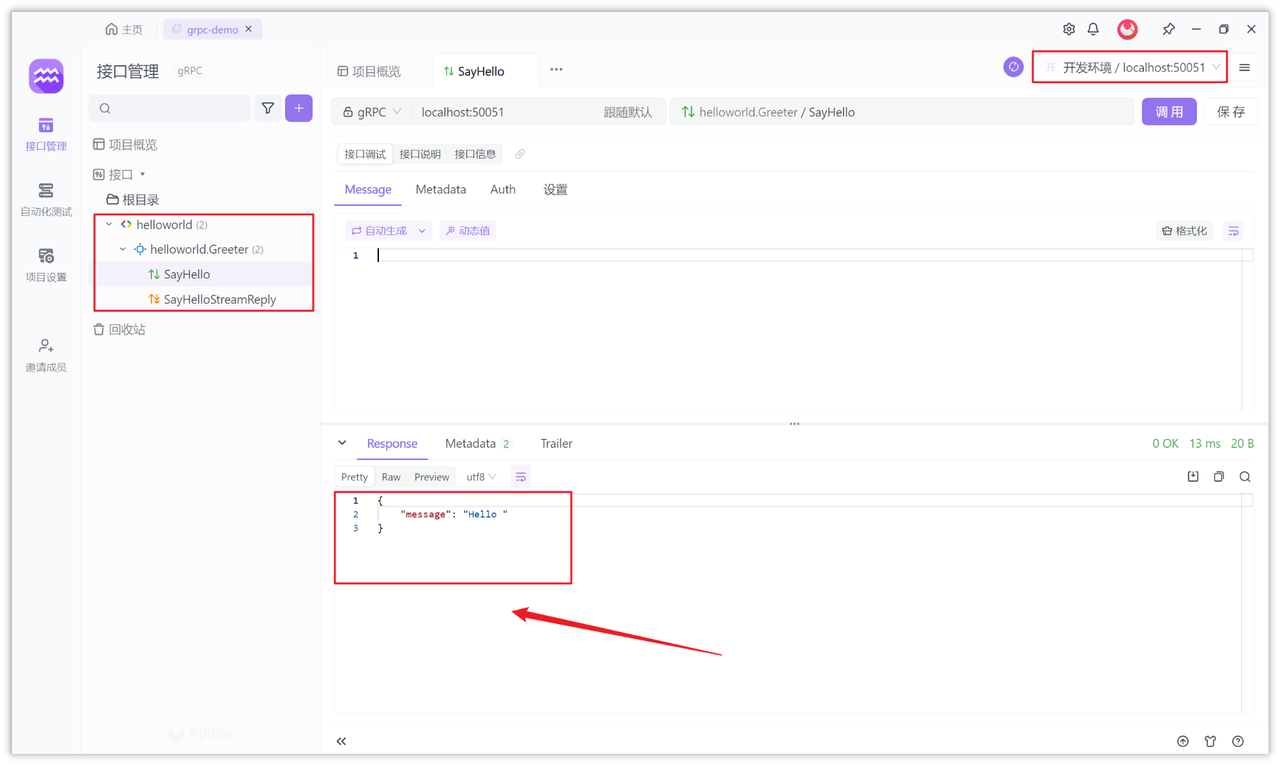
通过这些简单的步骤,你可以在 Apifox 中方便地管理和调试你的 gRPC 项目。这个功能非常强大,更具体的你可以访问 Apifox 的 gRPC 帮助文档,赶快去试试吧!
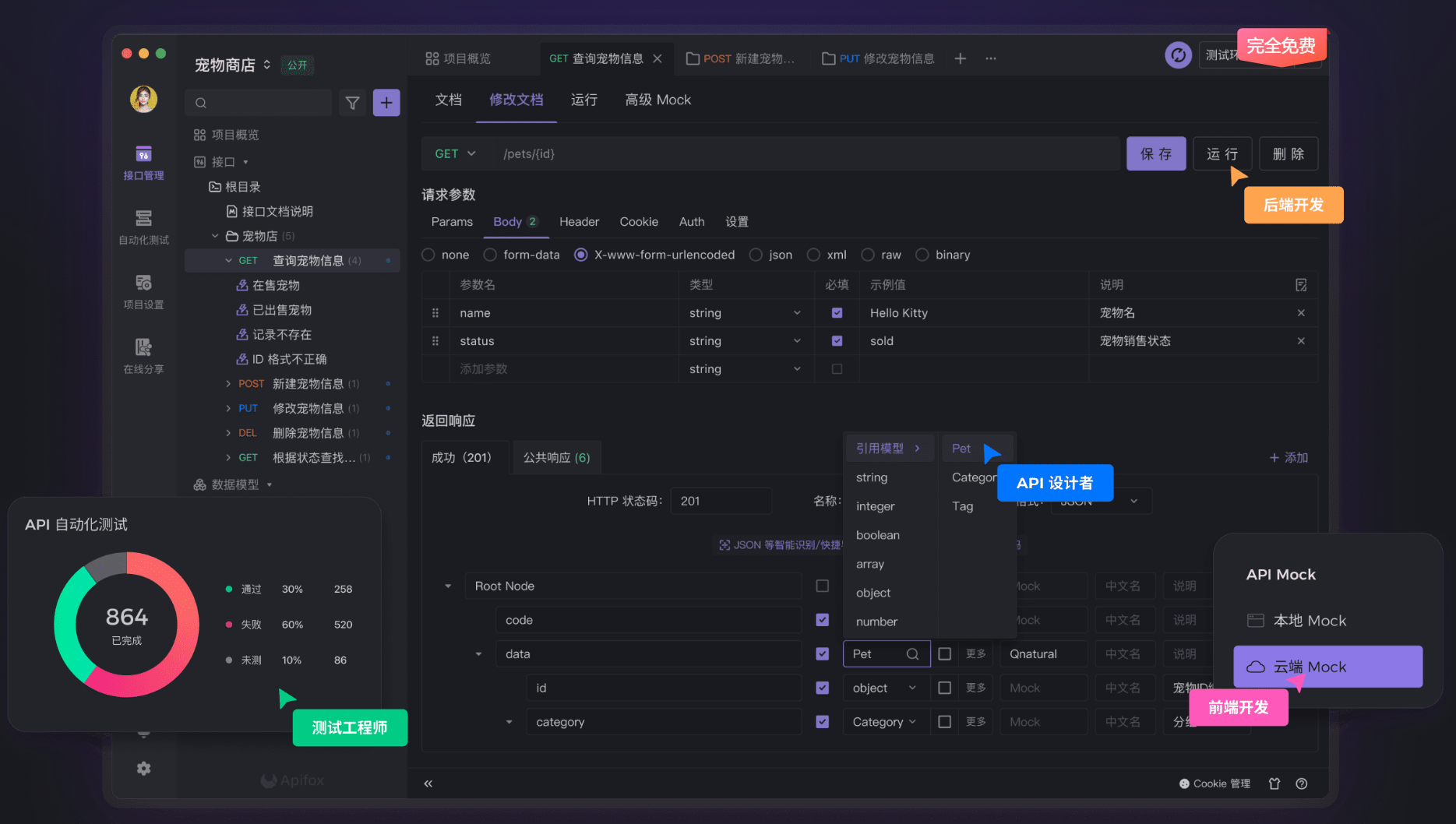
总结
以上说明了如何在一个新的 Java 项目中设置、实现和运行一个简单的 gRPC 服务。更多详情细节请参考官方文档,以及 gRPC Java GitHub 页面 上提供的更多示例和教程。
知识拓展:
参考连接:
- https://grpc.io/docs/languages/java/quickstart/
- https://github.com/grpc/grpc-java