在现代网络应用中,高性能和快速响应是至关重要的,Python 的 FastAPI 框架以其出色的性能和简单易用的特点,成为了许多开发者的首选。然而,在某些场景下,单线程运行可能无法满足需求,这时候就需要考虑使用多线程来提高应用的并发性能。本文将介绍 FastAPI 框架中多线程的使用方法,包括常见需求场景、问题解决方法以及实践案例。
常见需求场景
FastAPI多线程的使用在以下场景中特别有用:
- IO 密集型任务:当应用需要频繁进行文件读写、网络请求、数据库查询等耗时的 IO 操作时,使用多线程可以充分利用系统资源,提高响应速度。
- 并发处理:对于需要同时处理多个请求的场景,多线程可以实现并发处理,减少用户等待时间。
- 异步任务:在处理异步任务时,多线程可以使代码更加简洁高效。
常遇到的问题
在使用多线程时,需要注意以下常见问题:
- 线程安全问题:多线程共享资源时,可能会发生数据竞争等线程安全问题,需要通过锁或其他同步机制来避免。
- GIL(Global Interpreter Lock):Python 的 GIL 限制了同一进程中只有一个线程能够执行 Python 字节码,这意味着多线程并不能在多核处理器上实现真正的并行执行。对于 CPU 密集型任务,多线程并不是最佳选择。
使用多线程的方式
1.在路径操作函数中创建线程
可以直接在路径操作函数中使用 threading
模块创建线程。
import threading
from fastapi import FastAPI
app = FastAPI()
@app.get("/resource")
def get_resource():
t = threading.Thread(target=do_work)
t.start()
return {"message": "Thread started"}
def do_work():
# do computationally intensive work here
2.使用背景任务
FastAPI 提供了 @app.on_event("startup")
装饰器,可以在启动时创建后台任务。
from fastapi import FastAPI, BackgroundTasks
app = FastAPI()
@app.on_event("startup")
def startup_event():
threading.Thread(target=do_work, daemon=True).start()
def do_work():
while True:
# do background work
3.使用第三方后台任务库
可以使用第三方库如 apscheduler
来定期执行后台任务。
from apscheduler.schedulers.background import BackgroundScheduler
from fastapi import FastAPI
app = FastAPI()
scheduler = BackgroundScheduler()
@app.on_event("startup")
def start_background_processes():
scheduler.add_job(do_work, "interval", seconds=5)
scheduler.start()
def do_work():
# do periodic work
实践案例
安装所需工具
首先,确保已经安装了 Python 和 pip。然后,通过以下命令安装 FastAPI 和 uvicorn:
pip install fastapi
pip install uvicorn
编写多线程应用
我们来创建一个简单的 FastAPI 应用:
from fastapi import FastAPI
import threading
import time
app = FastAPI()
# 耗时的任务函数
def long_running_task(task_id: int):
print(f"Starting Task {task_id}")
time.sleep(5) # 模拟任务执行耗时
print(f"Finished Task {task_id}")
# 后台任务函数
def run_background_task(task_id: int):
thread = threading.Thread(target=long_running_task, args=(task_id,))
thread.start()
@app.get("/")
async def read_root():
return {"Hello": "World"}
@app.get("/task/{task_id}")
async def run_task_in_background(task_id: int):
# 创建并启动后台线程来运行任务
run_background_task(task_id)
return {"message": f"Task {task_id} is running in the background."}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="127.0.0.1", port=8000)
在这个示例中,我们使用 threading.Thread
类来创建一个后台线程来运行耗时的任务。run_background_task
函数中,我们创建了一个线程对象 thread
,并将 long_running_task
函数作为线程的 target
,并传递 task_id
作为参数。然后,我们调用 thread.start()
来启动新的线程,并在后台运行 long_running_task
。
运行应用
使用以下命令运行 FastAPI 应用:
uvicorn main:app --reload
然后,通过浏览器或工具如 curl 来测试应用。例如,打开浏览器,输入 http://localhost:8000/task/1
并按回车键。你将会立即收到响应 {"message": "Task 1 is running in the background."}
,并且在后台运行的任务将在5秒后打印出 "Finished Task 1"。期间,你可以继续发送其他任务请求。
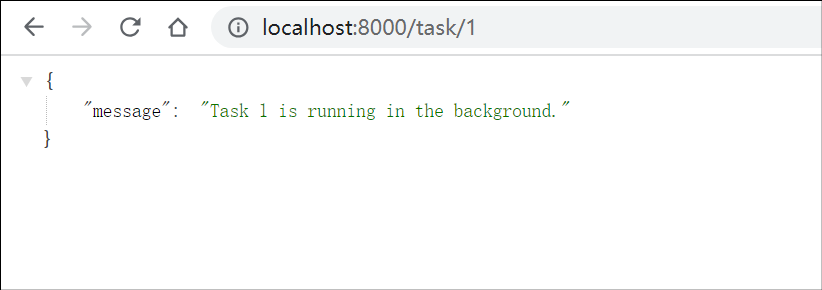
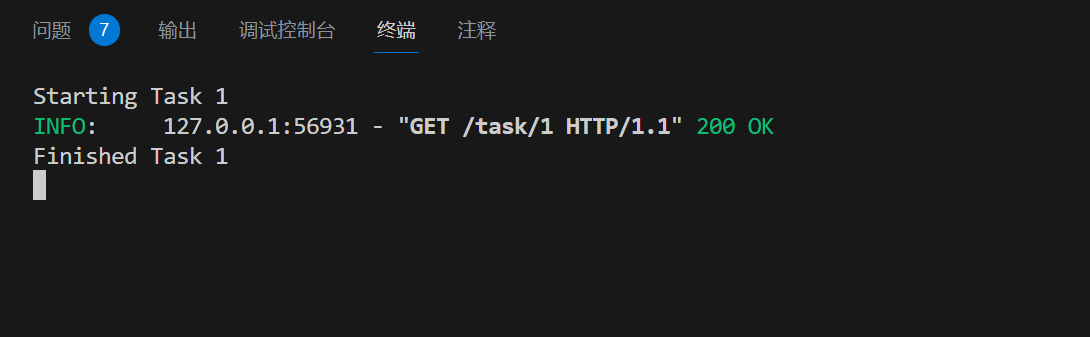
使用 Apifox 调试 FastAPI 接口
如果你是 FastAPI 开发者,你经常需要与 API 打交道,确保你的应用程序能够正常工作。这时,一个强大的接口测试工具就会派上用场。
Apifox 是一个集 API 文档、API 调试、API Mock 和 API 自动化测试于一体的 API 协作平台,我们可以通过 Apifox 来更方便的调试 FastAPI。
如果想快速的调试一条接口,新建一个项目后,在项目中选择“调试模式”,填写请求地址后即可快速发送请求,并获得响应结果,上文的实践案例如图所示:
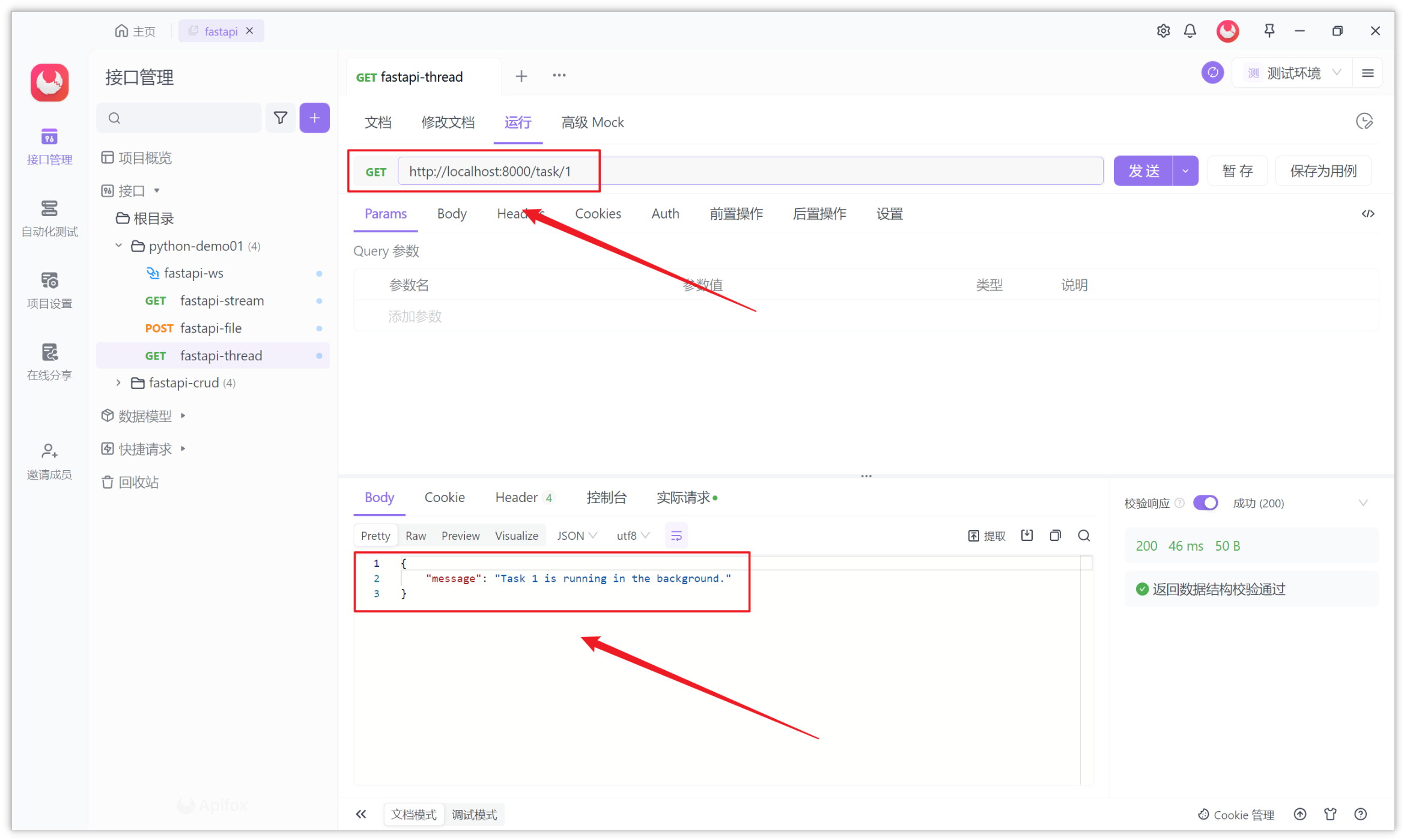
提示与注意事项
- 多线程并不适合 CPU 密集型任务,对于这类任务,可以考虑使用多进程(multiprocessing)来实现并行计算。
- 在使用多线程时,确保你的代码是线程安全的,避免数据竞争等问题。
- 如果你需要更高级的并发处理能力,可以尝试使用
asyncio
库来实现异步任务。
总结
本文介绍了 FastAPI 框架中多线程的使用方法。通过合理地应用多线程,我们可以提高应用的并发性能,让用户获得更好的体验。同时,我们还讨论了多线程可能遇到的问题,并给出了一些建议来避免这些问题。
知识扩展
了解更多 FastAPI 的知识:
参考链接
- FastAPI官方文档:https://fastapi.tiangolo.com/
- Python官方文档:https://docs.python.org/3/library/concurrent.futures.html