FastAPI 框架的灵活性和高性能让它成为很多开发者的心头好。尤其是当我们需要处理 API 请求时,DELETE 和 PUT 请求是两个重要的部分。DELETE 用于删除资源,而 PUT 则用来更新资源。让我们一起深入了解这两个请求的使用吧!
环境准备
我们需要确保我们的开发环境已经设置好。如果你还没有安装 FastAPI,可以通过下面的命令来安装:
pip install fastapi uvicorn
这里,我们还会安装 Uvicorn,这是一个用于运行 FastAPI 应用的 ASGI 服务器。
接下来,创建一个新的项目文件夹,并在其中建立一个 Python 文件,例如 main.py
。
创建 FastAPI 应用
我们需要初始化一个 FastAPI 应用。在 main.py
文件中,输入以下代码:
from fastapi import FastAPI
app = FastAPI()
这段代码就创建了一个基本的 FastAPI 应用。接下来,我们来设置路由,以便能够处理 PUT 和 DELETE 请求。
实现 PUT 请求
我们先来实现 PUT 请求。这是用于更新现有资源的。假设我们有一个用户资源,我们可以这样定义 PUT 路由:
from fastapi import Body
@app.put("/users/{user_id}")
async def update_user(user_id: int, user_data: dict = Body(...)):
return {"user_id": user_id, "updated_data": user_data}
在这里,我们使用了一个路径参数 user_id
和一个请求体 user_data
。当你发送 PUT 请求时,可以提供一个 JSON 格式的用户数据。比如:
{
"name": "Alice",
"age": 30
}
完整示例代码如下:
from fastapi import FastAPI, Body, HTTPException
app = FastAPI()
# 模拟一个用户数据库
fake_db = {
1: {"name": "Alice", "age": 30},
2: {"name": "Bob", "age": 25}
}
@app.put("/users/{user_id}")
async def update_user(user_id: int, user_data: dict = Body(...)):
if user_id not in fake_db:
raise HTTPException(status_code=404, detail="User not found")
fake_db[user_id].update(user_data)
return {"user_id": user_id, "updated_data": fake_db[user_id]}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="127.0.0.1", port=8000)
代码运行后,你可以通过 Apifox 等调试工具来调试这个 PUT 类型的接口,例如:http://127.0.0.1:8000/users/1
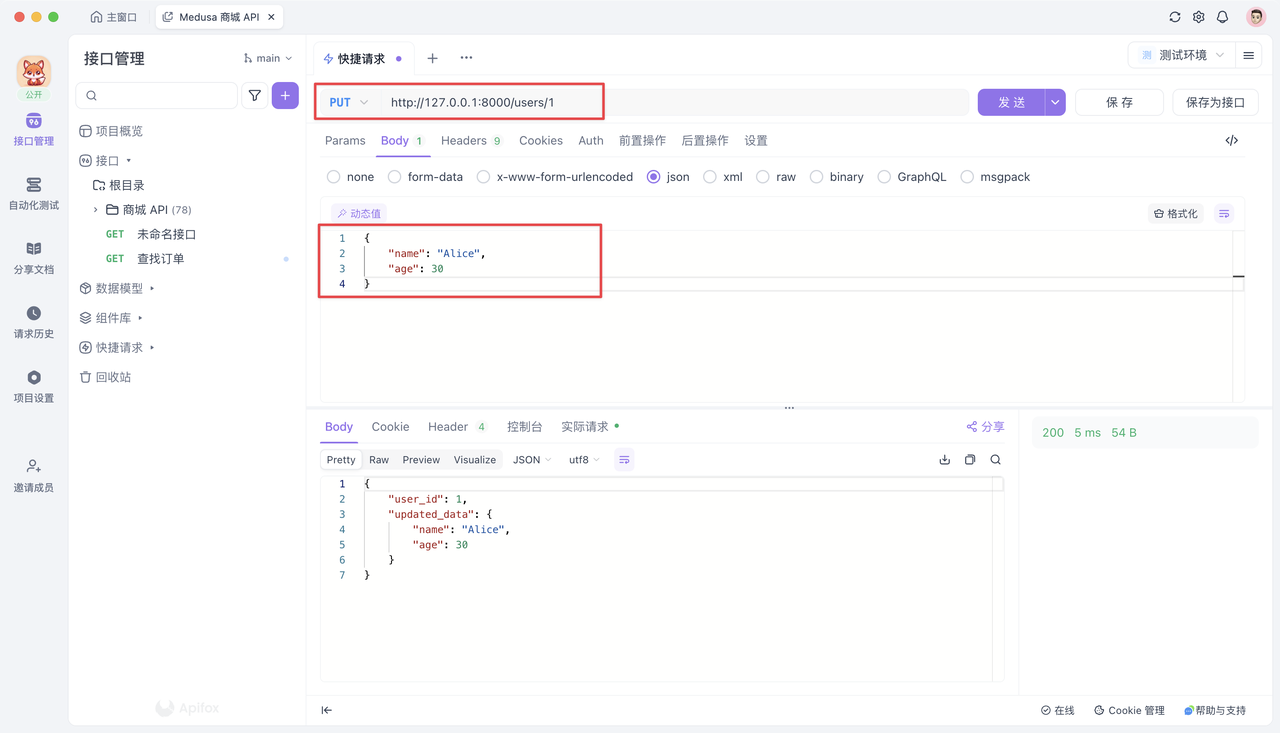
实现 DELETE 请求
现在我们来实现 DELETE 请求。这个请求是用来删除某个特定的资源。我们可以这样定义 DELETE 路由:
@app.delete("/users/{user_id}")
async def delete_user(user_id: int):
return {"message": f"User {user_id} deleted"}
这里,user_id
是我们要删除的用户的标识符。发送 DELETE 请求后,你将看到一个确认消息,告诉你该用户已被删除。
完整示例代码如下:
from fastapi import FastAPI, Body, HTTPException
app = FastAPI()
# 模拟一个用户数据库
fake_db = {
1: {"name": "Alice", "age": 30},
2: {"name": "Bob", "age": 25}
}
@app.delete("/users/{user_id}")
async def delete_user(user_id: int):
if user_id not in fake_db:
raise HTTPException(status_code=404, detail="User not found")
del fake_db[user_id]
return {"message": f"User {user_id} deleted"}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="127.0.0.1", port=8000)
代码运行后,你可以通过 Apifox 等调试工具来调试这个 DELETE 类型的接口,例如:http://127.0.0.1:8000/users/1
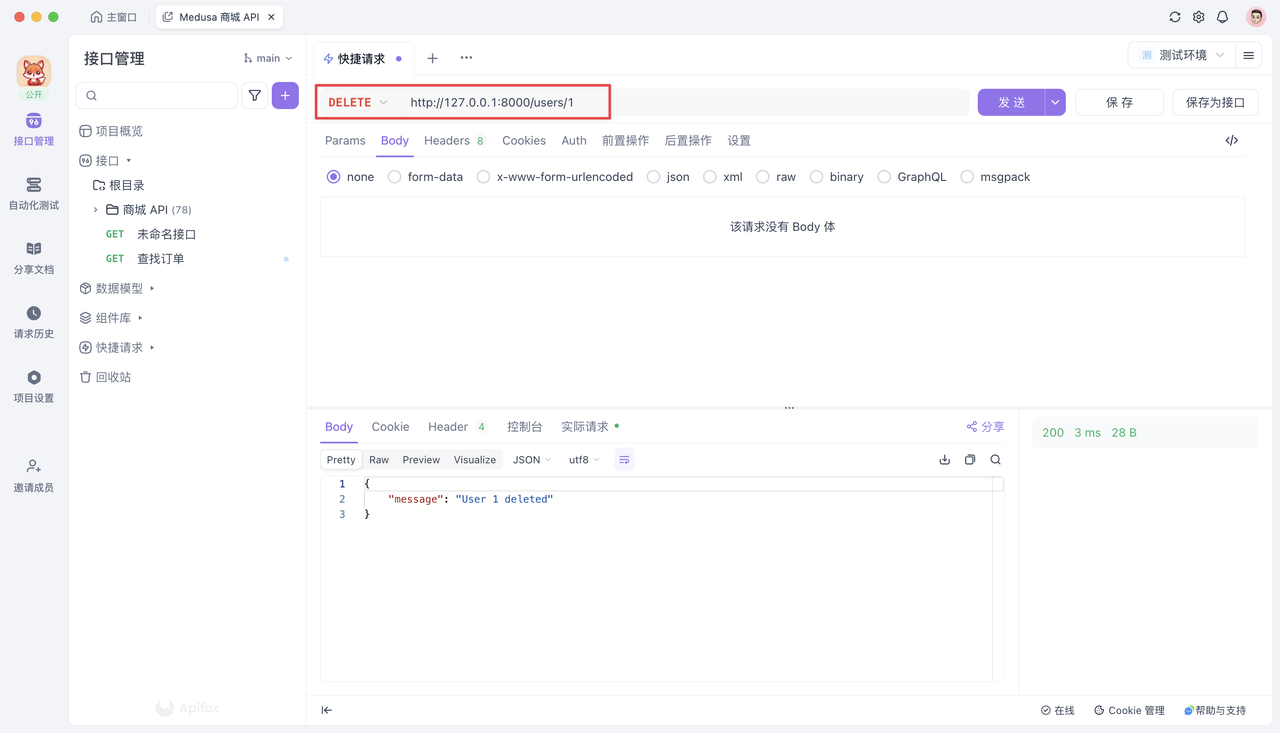
使用 Apifox 调试 PUT 和 DELETE 请求
Apifox 是一个非常实用的 API 文档和调试工具,它使得测试 API 请求变得简单明了。
步骤 1:创建项目
首先,打开 Apifox,创建一个新的 HTTP 项目。在项目中,你可以为不同的接口组织文件夹。
步骤 2:定义接口
添加 PUT 请求
- 点击“+”,然后选择“新建接口”。
- 输入接口名称,比如
更新用户
,选择方法为PUT
。 - 输入请求 URL,例如
http://127.0.0.1:8000/users/{user_id}
。 - 在请求体部分,选择
JSON
,并填入示例数据:
{
"name": "Alice",
"age": 30
}
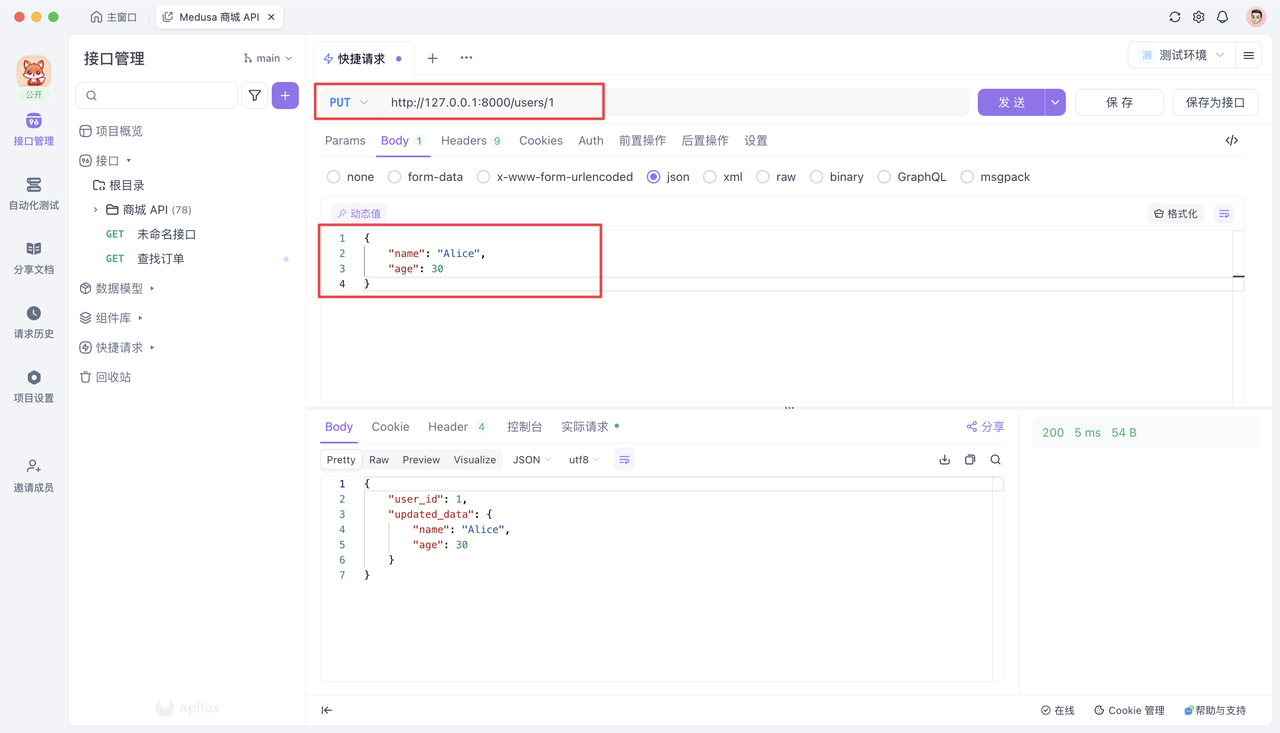
添加 DELETE 请求
- 同样的方法,创建一个新的接口,名称为
删除用户
,选择方法为DELETE
。 - 输入请求 URL,例如
http://127.0.0.1:8000/users/{user_id}
。
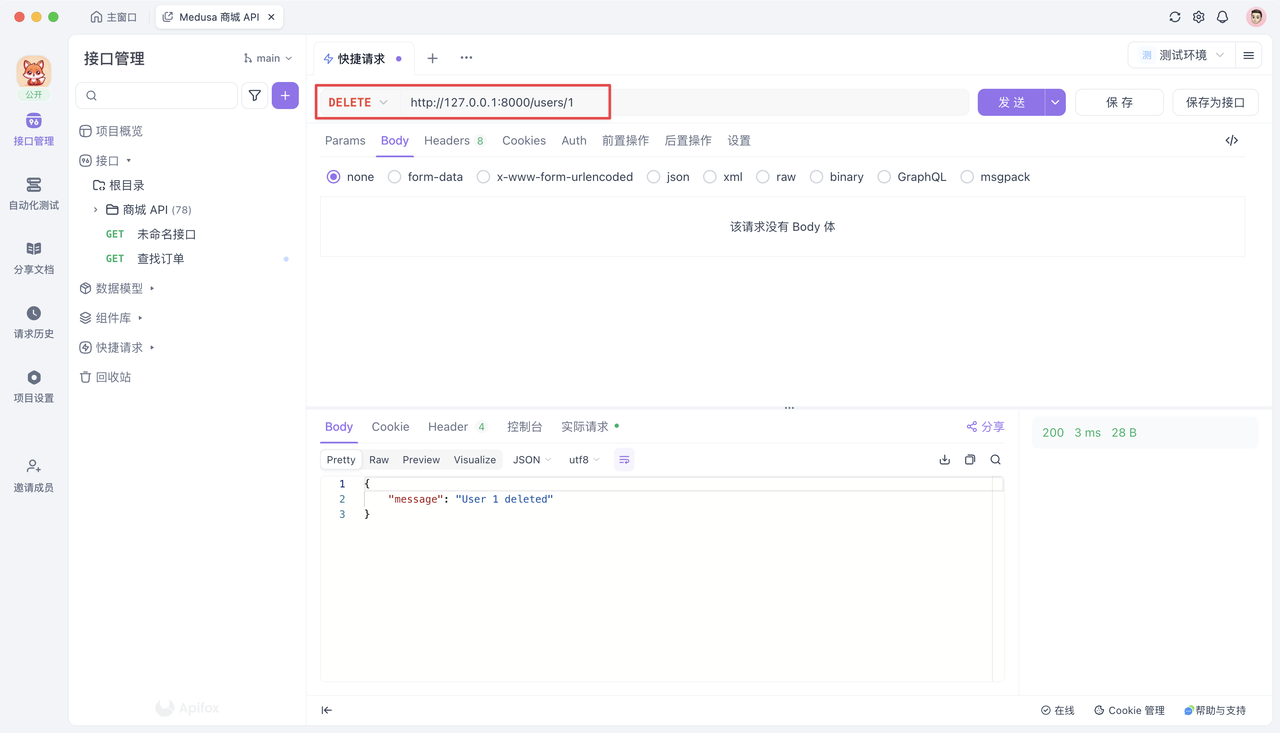
步骤 3:发送请求
在每个接口的页面上,点击“发送请求”按钮。Apifox 将会发送请求到你的 FastAPI 应用,并显示响应结果。
步骤 4:查看响应
你可以查看 API 响应的状态码、返回的 JSON 数据以及任何错误信息。这些信息将帮助你验证接口是否按照预期工作。
常见问题解答
在使用 PUT 和 DELETE 请求时,可能会遇到一些问题。比如,如果你尝试更新一个不存在的用户,FastAPI 会自动返回 404 错误。为了更好地处理这些错误,你可以在路由中加入错误处理逻辑。
总结
本文详细探讨了如何在 FastAPI 中使用 DELETE 和 PUT 请求,并展示了如何通过 Apifox 进行调试。这两个请求对于构建 RESTful API 非常重要。希望你能通过这个教程实践,并根据自己的需求扩展这些功能!
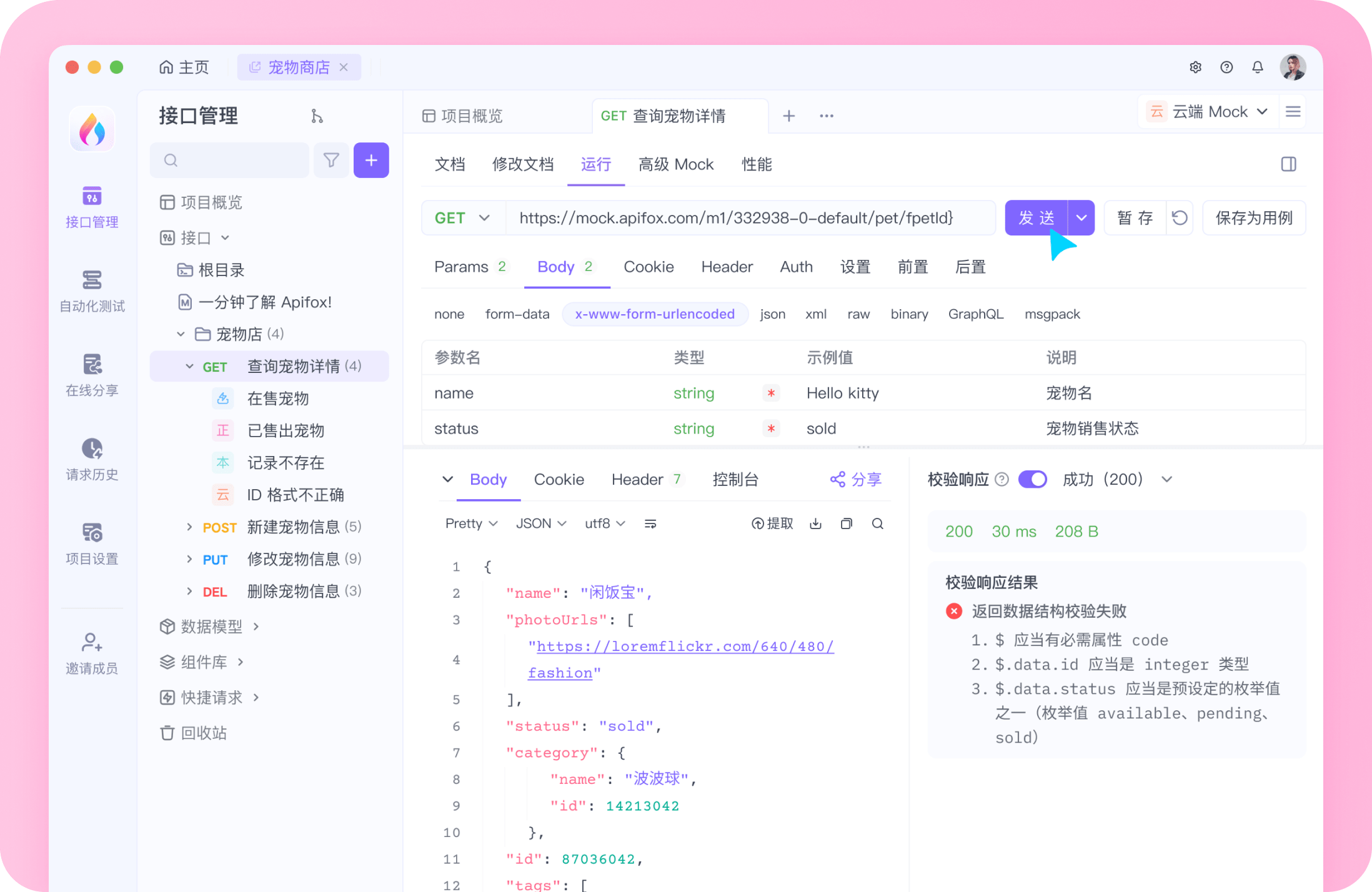